Navigating TypeScript's Type System: 24 Brilliant Advanced Techniques
27 February 20257 min read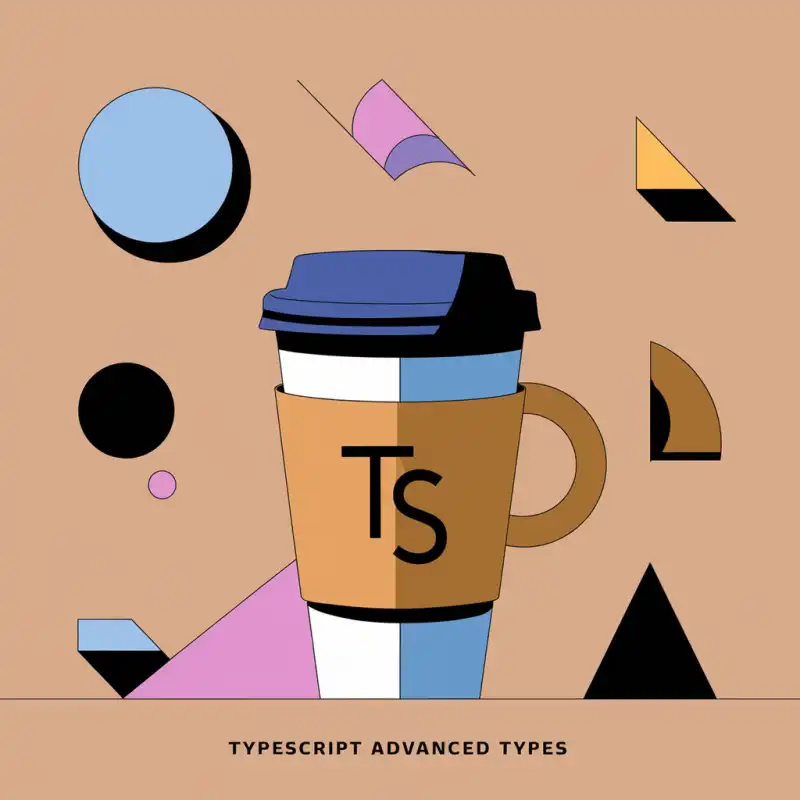
Diving deep into TypeScript’s type system is like navigating a labyrinth filled with elegant type inference, hidden traps, and mind-bending complexities. Whether you're a TypeScript beginner or a seasoned developer, these 24 advanced tips will help you conquer type challenges with precision and confidence.
1. Type Aliases vs. Interfaces: Know When to Use Each
When to Use
- Interfaces for defining object structures that require extension.
- Type aliases for unions, tuples, and function types.
1 interface User {2 id: string;3 avatarUrl: string;4 }56 type DeepPartial<T> = {7 [K in keyof T]?: T[K] extends object ? DeepPartial<T[K]> : T[K];8 };
🚫 Avoid: Using both on the same name. This causes conflicts.
1 interface DataType {2 name: string;3 }4 type DataType = { age: number }; // ❌ Error: Duplicate declaration
Summary
- Interfaces are best for merging and extending.
- Type aliases excel in complex operations.
2. Literal Type Locking: Preventing Accidental Errors
When to Use
- When restricting values to specific constants.
1 const routes = ["/home", "/about"] as const;2 function navigate(path: "/home" | "/about") {}34 navigate("/hom"); // ❌ Type error
🚫 Avoid: Using literal types when dynamic strings are needed.
Summary
- Literal types ensure accuracy but may reduce flexibility.
3. Type Assertions: Handle with Care!
When to Use
- When you are absolutely sure of a type.
1 const input = document.getElementById("search") as HTMLInputElement;
🚫 Avoid: Misusing assertions to force incorrect types.
1 const value = "42" as any as number; // ❌ Dangerous double assertion
Summary
- Type assertions are powerful but can lead to runtime errors if misused.
4. Type Compatibility: A Double-Edged Sword
When to Use
- When working with objects that share properties.
1 interface Point {2 x: number;3 y: number;4 }5 interface Vector {6 x: number;7 y: number;8 z: number;9 }1011 const printPoint = (p: Point) => {};12 const vector: Vector = { x: 1, y: 2, z: 3 };13 printPoint(vector); // ✅ Allowed but can be risky
🚫 Avoid: Relying on implicit compatibility for critical logic.
Summary
- Type compatibility allows flexibility but may lead to unexpected issues.
5. Type Guards: Safeguarding Your Code
When to Use
- When handling union types.
1 function isString(value: unknown): value is string {2 return typeof value === "string";3 }4 function format(input: string | number) {5 if (isString(input)) {6 return input.toUpperCase();7 }8 }
🚫 Avoid: Writing inaccurate type guards that can lead to false positives.
Summary
- Type guards enhance type safety but must be precise.
6. Utility Types: The TypeScript Swiss Army Knife
When to Use
- For reusable type transformations.
1 type ReadonlyUser = Readonly<User>;2 type PartialUser = Partial<User>;3 type DeepReadonly<T> = {4 readonly [K in keyof T]: T[K] extends object ? DeepReadonly<T[K]> : T[K];5 };
🚫 Avoid: Overcomplicating types, leading to degraded performance.
Summary
- Utility types reduce redundancy but should be used judiciously.
7. Function Overloading: Precise Control Over Inputs
When to Use
- For handling multiple input types cleanly.
1 function reverse(str: string): string;2 function reverse<T>(arr: T[]): T[];3 function reverse(value: any) {4 return typeof value === "string"5 ? value.split('').reverse().join('')6 : value.slice().reverse();7 }
🚫 Avoid: Placing a less specific overload before a more specific one.
Summary
- Function overloading ensures correctness but requires careful ordering.
8. Template Literal Types: Dynamic String Validation
When to Use
- For ensuring strict URL patterns.
1 type HttpMethod = "GET" | "POST" | "PUT" | "DELETE";2 type ApiRoute = `/api/${string}/${HttpMethod}`;34 const validRoute: ApiRoute = "/api/users/GET"; // ✅5 const invalidRoute: ApiRoute = "/api/posts/PATCH"; // ❌ Error
🚫 Avoid: Overusing them, leading to complex types.
Summary
- Template literal types enforce patterns but can get complicated.
9. Conditional Types: Smart Type Decisions
When to Use
- When types need to be evaluated dynamically.
1 type IsNumber<T> = T extends number ? true : false;2 type Check = IsNumber<42>; // true
🚫 Avoid: Excessive nesting, which degrades performance.
Summary
- Conditional types allow powerful logic but must be optimized.
10. Mapped Types: Efficient Batch Transformations
When to Use
- When modifying object properties.
1 type ReadonlyUser = {2 readonly [K in keyof User]: User[K];3 };
🚫 Avoid: Deeply nested mapped types that impact compilation time.
Summary
- Mapped types automate transformations but should be used cautiously.
11. Type Recursion Deep Waters
When to Use
- Handling infinitely nested data structures.
1 type Json = string | number | boolean | null | Json[] | { [key: string]: Json };23 const deepData: Json = {4 level1: {5 level2: [6 {7 level3: "Deep recursion warning!",8 },9 ],10 },11 };
⚠️ Avoid excessive depth to prevent compiler performance issues.
12. Index Access Types
When to Use
- Extracting deep types from objects.
1 type User = {2 profile: {3 name: string;4 age: number;5 };6 };78 type UserName = User["profile"]["name"]; // string
⛔ Be careful when accessing non-existent properties.
13. Conditional Distribution
When to Use
- Handling distribution characteristics of union types.
1 type StringArray<T> = T extends string ? T[] : never;2 type Result = StringArray<"a" | 1>; // "a"[]
⛔ Incorrect use may lead to type inference errors.
14. Type Guard
When to Use
- Creating custom type guards.
1 function isFish(pet: Fish | Bird): pet is Fish {2 return (pet as Fish).swim !== undefined;3 }
⛔ Ensure your guard logic is accurate to avoid false positives.
15. Discriminated Union
When to Use
- Handling structured yet distinct types.
1 type Shape =2 | { kind: "circle"; radius: number }3 | { kind: "square"; size: number };45 function area(s: Shape) {6 switch (s.kind) {7 case "circle":8 return Math.PI * s.radius ** 2;9 case "square":10 return s.size ** 2;11 default:12 throw new Error("Unknown shape");13 }14 }
✅ Ensures safer type narrowing.
16. Mutable Tuples
When to Use
- Handling flexible function parameters.
1 type Foo<T extends any[]> = [string, ...T, number];23 function bar(...args: Foo<[boolean]>) {}4 bar("test", true, 42); // ✅
⛔ Avoid overly long tuples as they impact type inference.
17. Type Query
When to Use
- Dynamically obtaining type information.
1 const user = { name: "Alice", age: 25 };2 type UserType = typeof user; // { name: string; age: number }
⛔ Excessive use can lead to type expansion.
18. Decorator Types
When to Use
- Applying type constraints to decorators.
1 function Log(target: any, key: string, descriptor: PropertyDescriptor): void {2 const original = descriptor.value as (...args: any[]) => any;3 descriptor.value = function (...args: any[]) {4 console.log(`Calling ${key} with`, args);5 return original.apply(this, args);6 };7 }
⛔ TypeScript decorators are experimental and may change in future updates.
19. Type Gymnastics
When to Use
- Simplifying complex type deductions.
1 type Simplify<T> = T extends infer U ? { [K in keyof U]: U[K] } : never;
⛔ Overusing complex type gymnastics can cause mental overload.
20. @ts-ignore
Usage
When to Use
- Bypassing type checking in emergencies.
1 // @ts-ignore2 const mystery: number = "42";
⚠️ Use sparingly, prefer @ts-expect-error
for better tracking.
21. Compilation Speed Optimization
When to Use
- Improving compilation times for large projects.
1 {2 "compilerOptions": {3 "skipLibCheck": true,4 "incremental": true,5 "tsBuildInfoFile": "./.tscache",6 "strict": true7 }8 }
⛔ Some checks may be skipped, maintain code quality!
22. Memory Leak Troubleshooting
When to Use
- Preventing type checking from consuming excessive memory.
1 type InfiniteRecursion<T> = {2 value: T;3 next: InfiniteRecursion<T>;4 };
⚠️ Limit recursion depth and avoid massive union types.
23. Type Versioning
When to Use
- Managing multiple versions of type definitions.
1 declare module "lib/v1" {2 interface Config {3 oldField: string;4 }5 }67 declare module "lib/v2" {8 interface Config {9 newField: number;10 }11 }
⚠️ Follow SemVer and handle cross-version types with caution.
24. Type Metaprogramming
When to Use
- Creating domain-specific type languages.
1 type ParseQuery<T extends string> =2 T extends `${infer K}=${infer V}&${infer Rest}`3 ? { [P in K]: V } & ParseQuery<Rest>4 : T extends `${infer K}=${infer V}`5 ? { [P in K]: V }6 : {};78 type Query = ParseQuery<"name=Alice&age=25">; // { name: 'Alice'; age: '25' }
⛔ High complexity and compilation overhead, use cautiously!
Conclusion: Mastering TypeScript's Advanced Type System
By applying these advanced TypeScript techniques, you can:
- Handle complex data structures efficiently.
- Improve type safety while balancing flexibility.
- Optimize compilation performance in large projects.
By mastering these advanced TypeScript techniques, you’ll be well-equipped to navigate the most challenging type-related scenarios with confidence. 🚀
Whenever you find yourself stuck in a TypeScript black hole, revisit this guide—it will light your path once more! 🔥