Introducing Hono: A Lightweight Backend Framework
5 September 20245 min read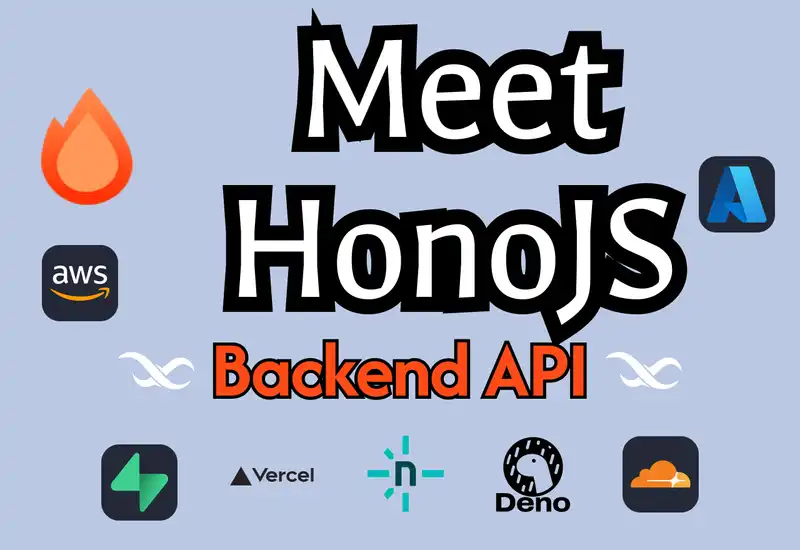
Hono is a cutting-edge, lightweight backend framework designed for cloud-native applications. Built on Node.js, Hono is optimized for speed and scalability, making it perfect for building high-performance APIs and services. Its minimalistic design allows developers to quickly create efficient, scalable solutions for modern cloud environments. Hono also offers support for edge computing, middleware, and routing, making it an excellent choice for developers looking to build cloud-native applications with minimal overhead and maximum performance.
Create a simple Backend
We will use Bun - an another JavaScript runtime, and Node.js-compatible package manager. If you are not familiar with Bun, please read this article.
1. Install Hono
1 ✔ Using target directory … hono-demo2 ? Which template do you want to use? bun3 ? Do you want to install project dependencies? yes4 ? Which package manager do you want to use? bun5 ✔ Cloning the template6 ✔ Installing project dependencies7 🎉 Copied project files8 Get started with: cd hono-demo
Move to hono-demo
cd hono-demoInstall dependencies
bun install2. Run the Hono app
Change the default port
1 // index.ts2 import { Hono } from "hono";34 const app = new Hono();56 app.get("/", (c) => {7 return c.text("Hello Hono!");8 });910 export default {11 port: 4200,12 fetch: app.fetch,13 };
Run the app
bun devResult:
1 $ bun run --hot src/index.ts2 Started server http://localhost:4200
Now the HTTP requests coming to the Bun server will be handled by Hono framework, providing us with a much more convenient API.
3. Grouped Routing in Hono.JS
According to the official Hono documentation, the framework supports grouped routing, allowing you to organize routes using an instance of Hono and add them to the main application using the route method.
Let’s create a post.ts inside a routes folder. Don’t forget to export posts as the default export:
1 // routes/post.ts2 import { Hono } from "hono";34 const post = new Hono();56 post.get("/", (c) => c.text("List Posts")); // GET /post7 post.get("/:id", (c) => {8 // GET /post/:id9 const id = c.req.param("id");10 return c.text("Get Post: " + id);11 });12 post.post("/", (c) => c.text("Create Book")); // POST /post1314 export default post;
Open Postman and navigate to http://localhost:4200/post/2 to test it
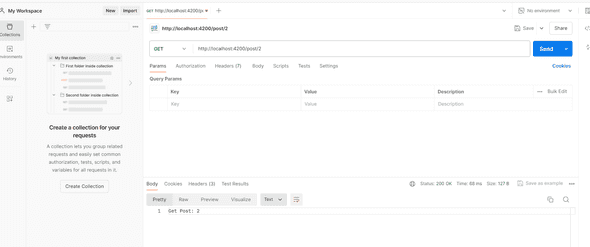
4. So, what exactly is the "c" in the arguments?
However, you might have noticed that c is used instead of req. This c stands for the context object, as detailed in the Hono.js documentation.
In practice, all incoming and outgoing data is managed by this context object. Hono allows you to return responses in various formats, not just JSON but also others, such as body, text, notFound, redirect and more.
Hono is also well-suited for handling and returning small amounts of HTML.
5. Rendering TSX/JSX
Although hono/jsx is typically used on the client side, it can also be utilized for server-side content rendering.
In the JSX section, there’s an example of a functional React component. Let’s try rendering it server-side:
Create a post page inside a pages folder:
1 // pages/post.tsx2 import { FC, PropsWithChildren } from "hono/jsx";34 type PostData = {5 title: string;6 id: string;7 };89 const Layout: FC = ({ children }: PropsWithChildren) => {10 return (11 <html>12 <body>{children}</body>13 </html>14 );15 };1617 const PostComponent = ({ title, id }: PostData) => {18 return (19 <Layout>20 <h1>Hello {title}</h1>21 <p>Your id is {id}</p>22 </Layout>23 );24 };2526 export default PostComponent;
Now Let’s connect it to our post route:
1 // routes/post.tsx23 post.get("/:id", (c) => {4 // GET /book/:id5 const id = c.req.param("id");6 return c.html(<PostComponent id={id} title={" World!"} />);7 });
Open http://localhost:4200/post/2 in your browser to test it.
This approach is similar to SSR in Next.js or Remix.js, but it's much lighter. Hono also supports other features like asynchronous components, Suspense, and more.
6. Middleware
Middleware is a function that integrates into the routing process and performs various operations.
You can intercept a request before it's processed or modify a response before it's sent.
HonoJS has a lot of built-in middleware, and you can add your own or reuse middleware created by the community.
Let's take a look at the official example from the documentation.
Add this code to index.ts file, before routes initialization
1 app.use(async (c, next) => {2 const start = Date.now();3 await next();4 const end = Date.now();5 c.res.headers.set("X-Response-Time", `${end - start}`);6 });
Check the result in Postman
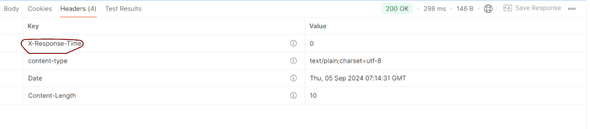
7. Handling redirects
You can create a redirect using the c.redirect() method:
1 c.redirect("/go-there");2 c.redirect("/go-there", 301);
8. Handling CORS
To enable our application to work with a frontend framework, we need to implement CORS. Hono offers a cors middleware for this purpose. Import and configure this middleware in your index.ts file:
1 import { cors } from "hono/cors";23 app.use("/api/*", cors());
9. Testing
Create index.test.ts file:
1 import { describe, expect, test } from "bun:test";2 import { Hono } from "hono";3 import { testClient } from "hono/testing";45 describe("Example", async () => {6 const app = new Hono().get("/", (c) => c.json("Hello Hono!"));7 const res = await testClient(app).$get();8 test("GET /post", async () => {9 const res = await app.request("/");10 expect(res.status).toBe(200);11 expect(await res.json()).toEqual("Hello Hono!");12 });13 });
Now run
bun test1 bun test v1.1.26 (0a37423b)23 src\index.test.ts:4 ✓ Example > GET /post56 1 pass7 0 fail8 2 expect() calls9 Ran 1 tests across 1 files. [301.00ms]
Conclusion
Creating a simple backend with Hono is both straightforward and efficient. With its lightweight design and built-in features, Hono allows you to quickly set up a server capable of handling a variety of tasks, from routing to middleware integration. By leveraging Hono's powerful capabilities, such as built-in support for CORS and flexible response formats, you can build robust backend solutions with minimal effort. Whether you’re developing a small-scale application or experimenting with new ideas, Hono provides the tools you need to get your project up and running smoothly.