How to Fix "__dirname is Not Defined" in NodeJs
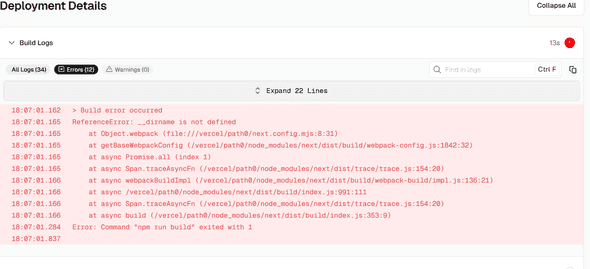
If you’re encountering the error __dirname is not defined in your Node.js project, it’s likely because you are using ES modules ("type": "module" in your package.json) instead of CommonJS modules. In ES modules, __dirname is not available by default. Here are some methods to fix it:
1. Manually Define __dirname
You can recreate the __dirname
functionality using import.meta.url
. Here’s how:
1 import { fileURLToPath } from 'url';2 import { dirname } from 'path';34 // Create __filename and __dirname5 const __filename = fileURLToPath(import.meta.url);6 const __dirname = dirname(__filename);78 console.log(__dirname); // Now __dirname works
This will give you the same behavior as __dirname
and __filename
in CommonJS.
2. Use CommonJS (Remove "type": "module"
)
If you don’t need ES modules, you can remove "type": "module"
from your package.json
file. This way, Node.js will use CommonJS, where __dirname
is natively supported.
3. Use a bundler (like Webpack or Rollup)
If you are working on a project that gets bundled for the browser (where __dirname
doesn’t exist), you can configure a bundler like Webpack to polyfill it:
- In Webpack, add this to your config:
1 node: {2 __dirname: false,3 }
This allows you to avoid the error in your browser-based projects.
By applying one of these methods, you can resolve the __dirname
issue and ensure that your project runs smoothly.