Using HTML5 Canvas to Add Watermarks to Images
Adding watermarks to images with HTML5 Canvas is straightforward and allows you to customize the placement, opacity, and styling of the watermark. Here’s a guide on how to add text or image watermarks using Canvas.
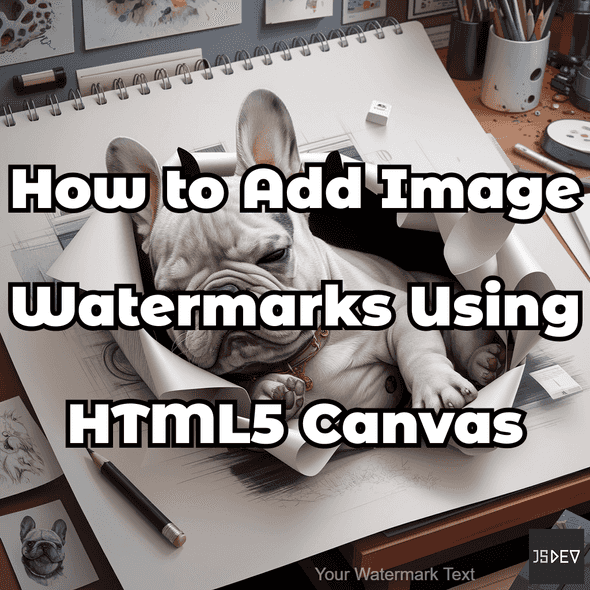
1. Set Up the HTML Structure
First, add an <img>
tag for the source image and a <canvas>
element in your HTML.
1 <!DOCTYPE html>2 <html lang="en">3 <head>4 <meta charset="UTF-8">5 <meta name="viewport" content="width=device-width, initial-scale=1.0">6 <title>Document</title>7 <link rel="stylesheet" href="style.css">8 </head>9 <body>10 <img id="sourceImage" src="buldog.png" alt="Buldog image" style="display:none;">11 <canvas id="canvas"></canvas>12 <script src="app.js"></script>13 </body>14 </html>
2. JavaScript Code for Adding a Text Watermark
You can use the Canvas API to draw the image and add a text watermark. Here’s how:
1 const canvas = document.getElementById('canvas');2 const context = canvas.getContext('2d');3 const image = document.getElementById('sourceImage');45 // Set the canvas size to match the image dimensions6 image.onload = function() {7 canvas.width = image.width;8 canvas.height = image.height;9 context.drawImage(image, 0, 0);1011 // Set text properties for the watermark12 context.font = "30px Arial";13 context.fillStyle = "rgba(255, 255, 255, 0.5)"; // white text with 50% opacity14 context.textAlign = "right";15 context.textBaseline = "bottom";1617 // Draw the watermark text18 context.fillText("Your Watermark Text", canvas.width - 10, canvas.height - 10); // bottom-right corner19 };
This code will display the image on the canvas, and then add semi-transparent text in the bottom-right corner.
3. Adding an Image Watermark
If you prefer using an image as a watermark, load the watermark image separately and draw it on top of the main image.
1 const watermarkImage = new Image();2 watermarkImage.src = 'path/to/watermark.png';34 watermarkImage.onload = function() {5 context.drawImage(watermarkImage, canvas.width - watermarkImage.width - 10, canvas.height - watermarkImage.height - 10);6 };
Here, watermarkImage
is drawn near the bottom-right corner of the canvas, 10 pixels from the edges. Adjust its opacity in an image editor beforehand or in Canvas using globalAlpha
:
1 context.globalAlpha = 0.5; // 50% opacity2 context.drawImage(watermarkImage, canvas.width - watermarkImage.width - 10, canvas.height - watermarkImage.height - 10);3 context.globalAlpha = 1.0; // Reset opacity for future drawings
4. Export the Result
To save or use the image with the watermark, convert the canvas content to a data URL:
1 const watermarkedImageURL = canvas.toDataURL("image/png");
You can use this data URL to download the watermarked image or set it as the src
for another <img>
tag.
Complete Code
Combining all steps into one:
1 <img id="sourceImage" src="path/to/your-image.jpg" alt="Source Image" style="display:none;">2 <canvas id="canvas"></canvas>34 <script>5 const canvas = document.getElementById('canvas');6 const context = canvas.getContext('2d');7 const image = document.getElementById('sourceImage');89 image.onload = function() {10 canvas.width = image.width;11 canvas.height = image.height;12 context.drawImage(image, 0, 0);1314 // Adding Text Watermark15 context.font = "30px Arial";16 context.fillStyle = "rgba(255, 255, 255, 0.5)";17 context.textAlign = "right";18 context.textBaseline = "bottom";19 context.fillText("Your Watermark Text", canvas.width - 10, canvas.height - 10);2021 // Adding Image Watermark22 const watermarkImage = new Image();23 watermarkImage.src = 'path/to/watermark.png';24 watermarkImage.onload = function() {25 context.globalAlpha = 0.5; // Set opacity26 context.drawImage(watermarkImage, canvas.width - watermarkImage.width - 10, canvas.height - watermarkImage.height - 10);27 context.globalAlpha = 1.0; // Reset opacity28 };29 };30 </script>
Customization Tips
- Adjust the position of the text and image watermarks by modifying the
x
andy
coordinates infillText
anddrawImage
. - Change
context.globalAlpha
to control the transparency of the image watermark. - Customize
context.font
andcontext.fillStyle
to style the text watermark to your liking.
This code allows you to overlay both text and image watermarks on your canvas, with adjustable styling options. You can further customize by changing font properties, color, and positioning.