Setting Up Node.js with Parcel and TypeScript
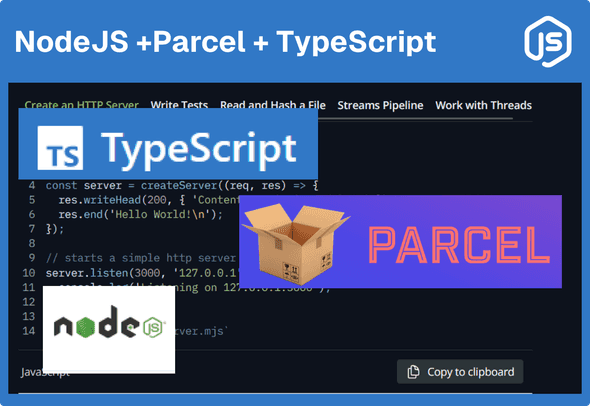
If you're looking to streamline your Node.js development process, Parcel and TypeScript are a great combination to ensure fast bundling and strong type-checking. This guide will walk you through setting up a Node.js project using Parcel as the bundler and TypeScript for type safety.
Step 1: Initialize Your Node.js Project
Start by creating a new directory for your project.
Initialize npm:
Run the following command to initialize a new Node.js project:
This will create a package.json file in your project directory.
Step 2: Install Parcel Bundler
Parcel is an easy-to-use bundler that requires minimal configuration.
Install Parcel:
To install Parcel as a development dependency, run:
Step 3: Set Up TypeScript
TypeScript adds static types to JavaScript, helping you catch errors early in development.
1. Install TypeScript and Node.js Type Definitions:
Run the following command to install TypeScript along with the Node.js type definitions:
2. Initialize TypeScript Configuration:
Create a tsconfig.json file by running:
3. Configure tsconfig.json:
Modify your tsconfig.json to include the following settings:
1 {2 "compilerOptions": {3 "target": "es6",4 "module": "commonjs",5 "strict": true,6 "esModuleInterop": true7 },8 "include": ["src/**/*"]9 }
Step 4: Create the Source Files
1. Create a src Directory:
Inside your project root, create a src folder where your TypeScript files will reside:
2. Add Your First TypeScript File:
Inside the src directory, create an index.ts file with some starter code:
Step 5: Configure Parcel for TypeScript
Parcel works out-of-the-box with TypeScript, but you need to define an entry point in your package.json.
1. Add Scripts to package.json:
Update the package.json file to include the following script:
1 "scripts": {2 "dev": "parcel src/index.html",3 "build": "parcel build src/index.html"4 }
If you're not using an HTML file, use parcel src/index.ts as the entry point.
Step 6: Run the Development Server
Start the Development Server:
Run Parcel’s development server with hot-reloading enabled:
Build for Production:
When you’re ready to bundle your code for production, run:
Conclusion
With Parcel and TypeScript set up, your Node.js project is ready for development. Parcel’s minimal configuration and TypeScript's type safety will make your development process faster and more efficient.