Optimizing Regex for Better Performance
Regular expressions (regex) are powerful tools for text matching, but they can cause performance issues if not optimized. This guide covers techniques to enhance regex efficiency and reduce resource usage.
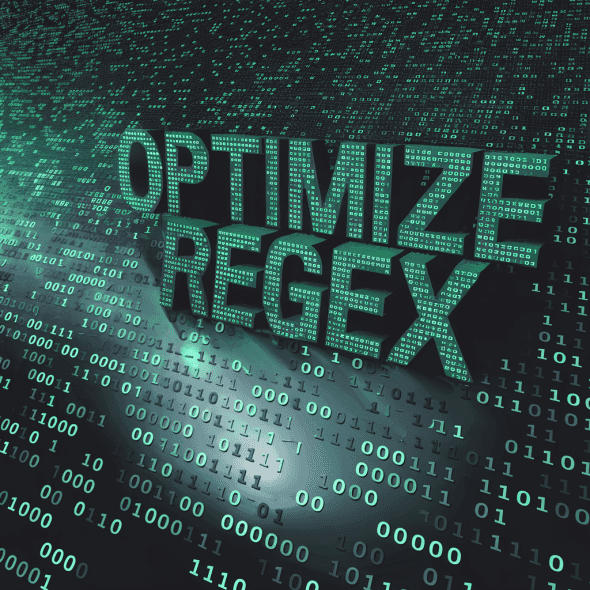
1. Use Anchors
Anchors (^
for start, $
for end) limit matching scope, reducing unnecessary attempts.
Example: To check if a string is numeric, use ^\d+$
Before Optimization:
1 const text = "abc123def456";2 const regex = /\d+/; // No anchor3 console.time("No Anchor Match");4 console.log(text.match(regex)); // [ '123' ]5 console.timeEnd("No Anchor Match");
After Optimization:
1 const regex = /^\d+$/; // With anchors2 console.time("Anchor Match");3 console.log(text.match(regex)); // null4 console.timeEnd("Anchor Match");
2. Use Lookaheads Judiciously
Positive ((?=...))
and negative ((?!...))
lookaheads enable conditional matching without consuming characters. Overuse may degrade performance.
Before Optimization:
1 const text = "123 word";2 const regex = /\d+\s\w+/; // Matches digits and word3 console.time("No Lookahead");4 console.log(text.match(regex)); // [ '123 word' ]5 console.timeEnd("No Lookahead");
After Optimization:
1 const regex = /\d+(?=\s\w+)/; // Matches digits followed by word2 console.time("Lookahead");3 console.log(text.match(regex)); // [ '123' ]4 console.timeEnd("Lookahead");
3. Compile Regular Expressions
For repeated use, compile regex to avoid re-parsing. In JavaScript, use new RegExp.
Before Optimization:
1 const regex = /abc\d+def/;2 console.time("Uncompiled Match");3 for (let i = 0; i < 1000; i++) regex.test("abc123def");4 console.timeEnd("Uncompiled Match");
After Optimization:
1 const regex = new RegExp("abc\\d+def"); // Compiled regex2 console.time("Compiled Match");3 for (let i = 0; i < 1000; i++) regex.test("abc123def");4 console.timeEnd("Compiled Match");
4. Use Non-Capturing Groups
Replace capturing groups ((...))
with non-capturing ones ((?:...))
to save resources.
Before Optimization:
1 const regex = /(abc|def)/; // Capturing group2 console.time("Capturing Group Match");3 console.log("abc or def".match(regex)); // ['abc', 'abc']4 console.timeEnd("Capturing Group Match");
After Optimization:
1 const regex = /(?:abc|def)/; // Non-capturing group2 console.time("Non-Capturing Group Match");3 console.log("abc or def".match(regex)); // ['abc']4 console.timeEnd("Non-Capturing Group Match");
Summary
Optimizing regex improves accuracy and performance, especially when processing large datasets. By implementing these techniques, you can create faster, more efficient regex tailored to specific needs.