Mastering React Performance Analysis
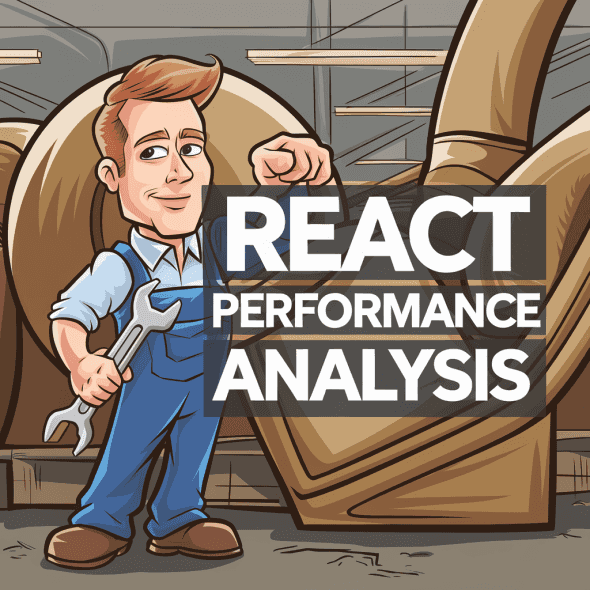
React applications can sometimes experience performance issues due to unnecessary re-renders and inefficient state management. Fortunately, there are several tools to help developers analyze and optimize React component performance. This article will cover three essential tools: React Profiler, WhyDidYouRender, and useTrackedEffect, providing insight into how they work and how to integrate them into your project.
1. React Profiler: Measuring Component Rendering Performance
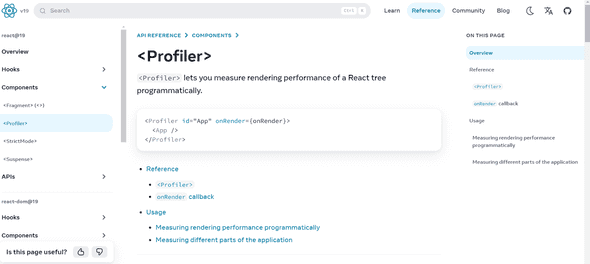
The React Profiler is an in-built tool that helps developers measure the performance of React applications. It tracks the rendering time of components, the frequency of re-renders, and the cost associated with rendering, enabling developers to identify performance bottlenecks.
To use the React Profiler, wrap your component tree with the <Profiler>
component:
1 <Profiler id="App" onRender={onRender}>2 <App />3 </Profiler>
onRender
is a callback function that React calls whenever the component tree is updated. It provides the following parameters:
- id: Identifies the part of the UI being measured.
- phase: Indicates if the component is mounting or updating.
- actualDuration: Time taken to render the component.
- baseDuration: Estimated time to re-render the subtree without optimizations.
- startTime: Timestamp when rendering started.
- commitTime: Timestamp when rendering was committed.
Use actualDuration
to identify when a component takes too long to render. If it exceeds 16ms, it may indicate a performance issue.
2. WhyDidYouRender: Understanding Unnecessary Re-Renders
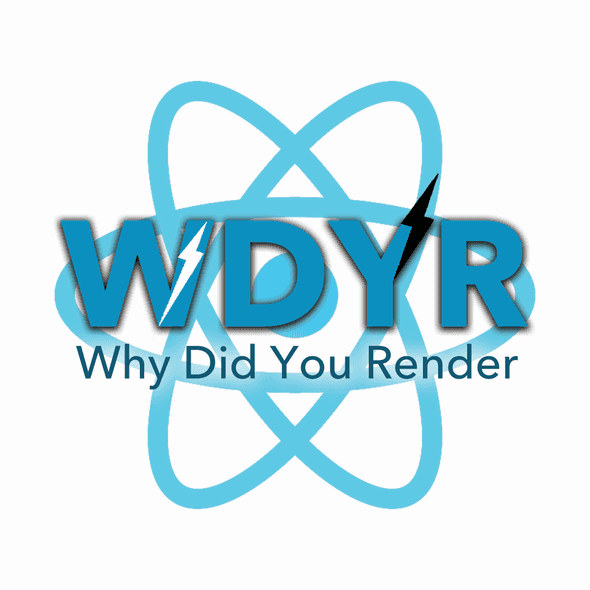
WhyDidYouRender is a utility that helps track unnecessary re-renders in React components. It provides detailed information about why a component re-rendered, including which props or state changes triggered the re-render. This tool is especially helpful for optimizing performance when a component is unnecessarily re-rendered.
To integrate WhyDidYouRender into a Vite project:
1. Install the package:
2. Modify your vite.config.js
:
1 export default defineConfig({2 plugins: [3 react({4 jsxImportSource: "@welldone-software/why-did-you-render",5 }),6 ],7 });
3. Create a wdyr.js
file:
1 import React from "react";2 import whyDidYouRender from "@welldone-software/why-did-you-render";34 whyDidYouRender(React, {5 trackAllPureComponents: true,6 trackHooks: true,7 collapseGroups: true,8 });
4. Import the wdyr.js
file in main.jsx
:
1 import './wdyr.js';
Key configuration options in WhyDidYouRender:
include
: Specifies which components to trace based on a regular expression.trackHooks
: If enabled, tracks all React Hook changes.logOwnerReasons
: Logs the parent component that caused the re-render.
For a component to trigger logging, set whyDidYouRender
to true:
1 Component.whyDidYouRender = true;
3. useTrackedEffect: Efficiently Handling State Changes
The useTrackedEffect hook is designed to track state changes and execute side effects only when necessary, improving performance over the standard useEffect
.
Example:
1 import React, { useState } from 'react';2 import { useTrackedEffect } from '../hooks';34 export default () => {5 const [count, setCount] = useState(0);6 const [count2, setCount2] = useState(0);78 useTrackedEffect(9 (changes) => {10 console.log('Changed dependencies: ', changes);11 },12 [count, count2],13 );1415 return (16 <div>17 <p>Count: {count}</p>18 <button onClick={() => setCount(count + 1)}>Increment</button>19 <p>Count2: {count2}</p>20 <button onClick={() => setCount2(count2 + 1)}>Increment</button>21 </div>22 );23 };
In the above example, useTrackedEffect
detects which dependencies (e.g., count
or count2
) have changed and logs the changes, helping avoid unnecessary side effects.
Conclusion
By utilizing these three tools—React Profiler, WhyDidYouRender, and useTrackedEffect—you can optimize the performance of your React components. React Profiler helps you measure rendering performance, WhyDidYouRender identifies unnecessary re-renders, and useTrackedEffect
helps efficiently track state changes. Integrating these tools into your development workflow can significantly improve the performance and efficiency of your React applications.