Implementing Lazy Loading in React
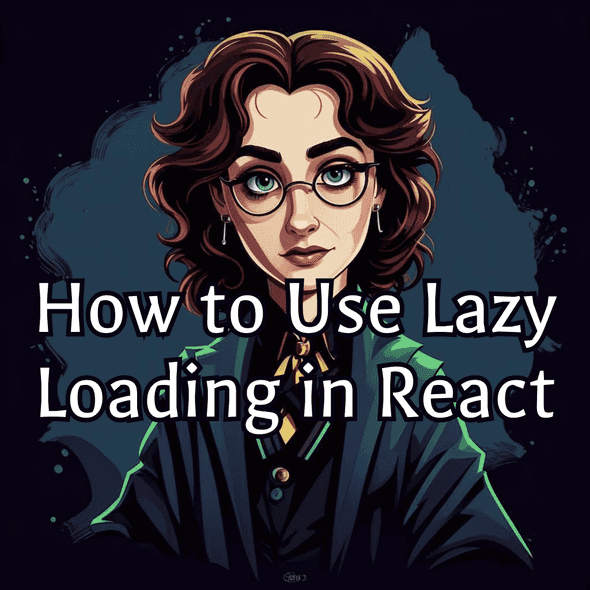
React lazy loading allows components to be loaded only when they are needed, improving the performance of your application by reducing the initial bundle size. It’s especially useful for optimizing larger applications where you don’t want to load everything upfront.
Here’s a step-by-step guide on how to implement lazy loading in React:
1. Using React.lazy()
React provides a built-in function, React.lazy(), that lets you dynamically import a component. This method tells React to only load the component when it’s actually rendered.
Example:
1 import React, { Suspense } from 'react';23 const LazyComponent = React.lazy(() => import('./LazyComponent'));45 function App() {6 return (7 <div>8 <h1>Main App</h1>9 <Suspense fallback={<div>Loading...</div>}>10 <LazyComponent />11 </Suspense>12 </div>13 );14 }1516 export default App;
- ** React.lazy(() => import('./LazyComponent'))** dynamically imports the LazyComponent.
- The Suspense component is used to show a fallback (like a loading spinner) while the lazy component is loading.
2. Using Suspense for Fallback
The Suspense component is crucial for lazy loading because it provides a fallback UI while waiting for the lazy-loaded component. The fallback prop allows you to define what will be displayed while the component is being loaded, such as a loading spinner or text.
1 <Suspense fallback={<div>Loading component...</div>}>2 <LazyComponent />3 </Suspense>
Without the Suspense component, React will not know how to handle the loading state, and it will throw an error.
3. Lazy Loading Routes
Lazy loading is especially useful when working with routes in React. By lazily loading route components, you can reduce the amount of code that needs to be loaded upfront, speeding up the initial render.
Example with React Router:
1 import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';2 import React, { Suspense } from 'react';34 const Home = React.lazy(() => import('./Home'));5 const About = React.lazy(() => import('./About'));67 function App() {8 return (9 <Router>10 <Suspense fallback={<div>Loading...</div>}>11 <Switch>12 <Route exact path='/' component={Home} />13 <Route path='/about' component={About} />14 </Switch>15 </Suspense>16 </Router>17 );18 }1920 export default App;
In this example:
- The Home and About components are lazily loaded when their respective routes are accessed.
- The fallback UI is displayed while the components are being loaded.
4. Code Splitting
React lazy loading works hand-in-hand with code splitting. By splitting the code into smaller bundles and only loading them when needed, you can significantly improve your app’s performance.
Webpack automatically supports code splitting with import() syntax, and using React.lazy() enables this in your React applications.
5. Error Boundaries for Lazy Components
If your lazy-loaded component fails to load due to network issues or other problems, you should handle these errors using Error Boundaries. Error boundaries are React components that catch JavaScript errors anywhere in the component tree.
Example:
1 class ErrorBoundary extends React.Component {2 constructor(props) {3 super(props);4 this.state = { hasError: false };5 }67 static getDerivedStateFromError() {8 return { hasError: true };9 }1011 render() {12 if (this.state.hasError) {13 return <h1>Something went wrong!</h1>;14 }15 return this.props.children;16 }17 }1819 // Wrap the Suspense component with ErrorBoundary20 <ErrorBoundary>21 <Suspense fallback={<div>Loading...</div>}>22 <LazyComponent />23 </Suspense>24 </ErrorBoundary>;
Conclusion
React lazy loading, along with Suspense, allows you to optimize your application by loading components only when they are needed. By using lazy loading, especially for routes and larger components, you can significantly reduce the initial bundle size, speeding up your app's load time and enhancing the user experience.