Using Decorators to Serialize Entities in TypeScript
Serialization is the process of converting an object into a format that can be easily stored or transmitted and later reconstructed. In TypeScript, decorators provide a powerful way to modify or annotate classes and their members. By combining decorators with serialization, you can streamline the process of converting complex objects into JSON or other formats. This article will guide you through the steps to serialize entities using decorators in TypeScript.
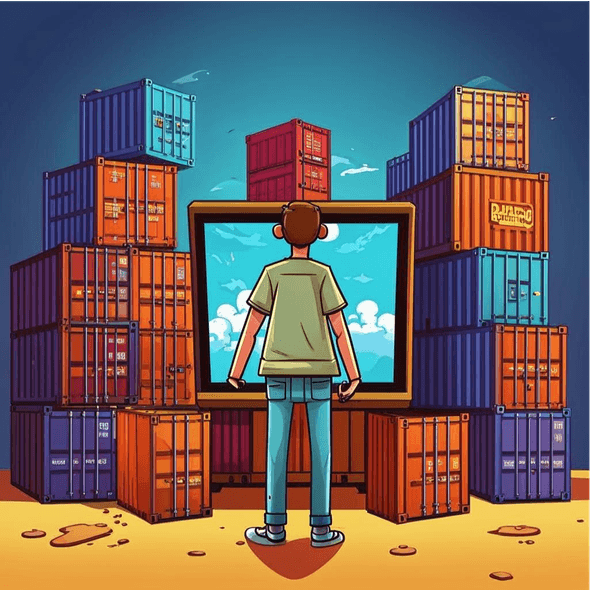
Step 1: Setting Up TypeScript with Decorators
First, make sure you have TypeScript set up with experimental decorators enabled, as decorators are still an experimental feature.
- Install TypeScript (if you haven't already):
- Enable decorators in your tsconfig.json:
1 {2 "compilerOptions": {3 "experimentalDecorators": true,4 "emitDecoratorMetadata": true5 }6 }
Step 2: Understanding Decorators
Decorators are special functions that can be attached to classes, properties, methods, or parameters. In the context of serialization, they allow you to control how a class or its properties are serialized into a specific format (like JSON).
Decorators in TypeScript can be created using the @
symbol followed by a function that takes
parameters related to the object being decorated.
Step 3: Creating a Serialization Decorator
Let's start by creating a simple decorator that will mark specific class properties for serialization.
1 function Serializable(target: any, key: string) {2 if (!target.constructor.serializableProperties) {3 target.constructor.serializableProperties = [];4 }5 target.constructor.serializableProperties.push(key);6 }
Here, the Serializable decorator is used to mark properties in a class for serialization. The target is the class, and key is the property name. We store all serializable properties in an array attached to the class's constructor.
Step 4: Applying the Decorator to a Class
Next, we create a class with properties marked for serialization using the @Serializable decorator.
1 class User {2 @Serializable3 public firstName: string;45 @Serializable6 public lastName: string;78 public password: string; // We don't want to serialize this910 constructor(firstName: string, lastName: string, password: string) {11 this.firstName = firstName;12 this.lastName = lastName;13 this.password = password;14 }15 }
In this example, the firstName and lastName properties are marked for serialization, but the password field is excluded since we don't apply the @Serializable decorator to it.
Step 5: Implementing the Serialization Logic
Now, let's add a function to serialize only the marked properties into JSON format.
1 function serialize(instance: any): string {2 const serializedData: any = {};3 const serializableProperties =4 instance.constructor.serializableProperties || [];56 serializableProperties.forEach((key: string) => {7 serializedData[key] = instance[key];8 });910 return JSON.stringify(serializedData);11 }
The serialize
function checks the class for properties that have been marked as serializable,
extracts their values, and converts them into a JSON string.
Step 6: Serializing the Object
Finally, let’s serialize a User object and see how it works:
1 const user = new User("John", "Doe", "secretPassword");2 const serializedUser = serialize(user);34 console.log(serializedUser); // Output: {"firstName":"John","lastName":"Doe"}
As you can see, the password field is not included in the serialized output because it wasn’t decorated with @Serializable.
Step 7: Extending the Decorator for Advanced Serialization
You can enhance the Serializable
decorator to handle more advanced cases, such as renaming
properties, setting custom rules, or handling nested objects. For instance, you can modify the
decorator to accept additional metadata:
1 function Serializable(alias?: string) {2 return function (target: any, key: string) {3 if (!target.constructor.serializableProperties) {4 target.constructor.serializableProperties = [];5 }6 target.constructor.serializableProperties.push({7 key,8 alias: alias || key,9 });10 };11 }
This would allow you to rename properties during serialization:
1 class User {2 @Serializable('first_name')3 public firstName: string;45 @Serializable('last_name')6 public lastName: string;78 public password: string;9 }
And the resulting JSON output will now have the renamed properties:
1 const user = new User("John", "Doe", "secretPassword");2 const serializedUser = serialize(user);34 console.log(serializedUser); // Output: {"first_name":"John","last_name":"Doe"}
Conclusion
Decorators in TypeScript offer a powerful and flexible way to handle serialization by allowing you to control which properties of an object should be serialized. With this approach, you can easily exclude sensitive data, rename properties, and even extend the functionality to handle complex nested structures.
By combining the power of decorators and serialization, you can ensure that your application's data is securely and efficiently handled when converting objects to and from formats like JSON.