How to Get Started with Zustand V5 for React State Management
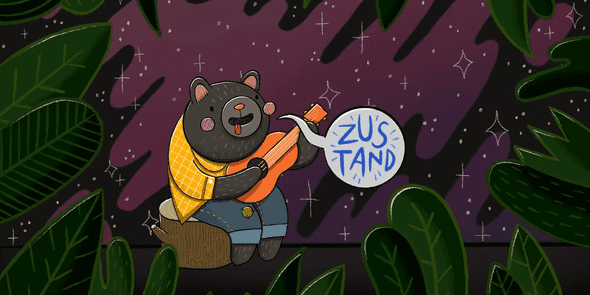
Zustand is a high-performance React state management library that simplifies state handling with minimal boilerplate code. Unlike Redux or MobX, Zustand eliminates the need for complex reducers, actions, or observable objects. Zustand V5 introduces notable improvements, leveraging React 18’s useSyncExternalStore
for optimized performance and a reduced package size.
In this comprehensive guide, we’ll explore Zustand V5’s key features, how to use it effectively, and the principles behind its design.
Key Features of Zustand
- Lightweight and Fast: Minimal API, optimized for performance, and only ~3KB.
- Selective Rendering: Updates only components that depend on the changed state.
- Flexible Middleware: Supports plugins like persist for data persistence.
- Simplified Usage: No complex setup; just define and use your store.
Getting Started with Zustand V5
Install Zustand in your project:
Create a Store
Define a simple store with state variables and actions:
1 import { create } from 'zustand';23 type Store = {4 count: number;5 increment: () => void;6 };78 const useStore = create<Store>((set) => ({9 count: 0,10 increment: () => set((state) => ({ count: state.count + 1 })),11 }));
Access State and Actions
Use the store in your components:
1 const Counter = () => {2 const count = useStore((state) => state.count);3 const increment = useStore((state) => state.increment);45 return (6 <div>7 <p>Count: {count}</p>8 <button onClick={increment}>Increment</button>9 </div>10 );11 };
Selective Rendering
Zustand optimizes performance by only re-rendering components subscribed to updated parts of the store. For example:
1 const DisplayCount = () => {2 const count = useStore((state) => state.count);3 return <p>Count: {count}</p>;4 };
Here, only DisplayCount
will re-render when count
changes.
Advanced Features
Middleware: Adding Persistence
Zustand supports middleware like persist to save data between sessions:
1 import { create } from 'zustand';2 import { persist } from 'zustand/middleware';34 const usePersistentStore = create(5 persist(6 (set) => ({7 count: 0,8 increment: () => set((state) => ({ count: state.count + 1 })),9 }),10 { name: 'counter-storage' }11 )12 );
Handling Complex Selectors
For optimized updates, use shallow comparisons or selector functions:
1 import { shallow } from 'zustand/shallow';23 const countData = useStore(4 (state) => ({ count1: state.count1, count2: state.count2 }),5 shallow6 );
This prevents unnecessary re-renders when unrelated state changes occur.
Internal Mechanisms
Zustand V5 utilizes React 18’s useSyncExternalStore
for efficient state synchronization. This ensures:
- Accurate Subscriptions: Only subscribed components update.
- Lightweight Logic: Directly interacts with React’s rendering process.
- Improved Performance: Reduced package size and faster reactivity.
Why Choose Zustand?
- Minimal Boilerplate: Simple API for quick integration.
- Performance-Oriented: Leverages React’s latest features for efficiency.
- Versatile Middleware: Extend functionality as needed.
- Perfect for Prototyping and Production: Lightweight yet powerful.
Conclusion
Zustand V5 is a game-changer for React developers seeking a state management tool that is both powerful and easy to use. Its minimalistic design, optimized performance, and flexible middleware make it a top choice for modern applications.
Start using Zustand V5 today to simplify your state management workflow and enhance your React projects.