Building a 3D Interactive Earth with Three.js
23 January 20254 min read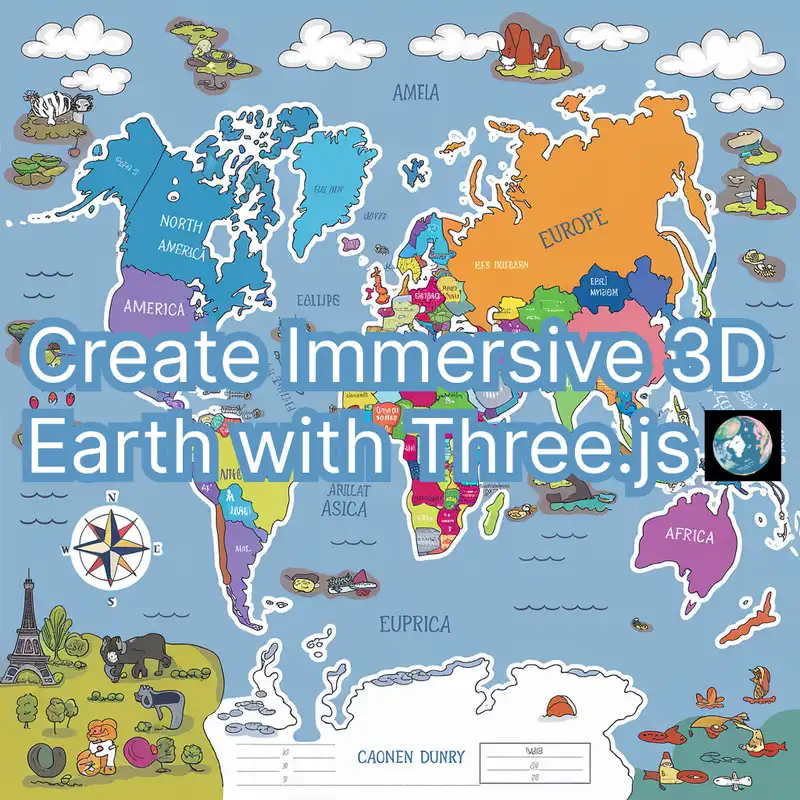
In modern web development, visual interaction is key to capturing user attention. Three.js, as one of the most powerful WebGL libraries, makes complex 3D visualization accessible. This article will detail how to create an interactive 3D earth display using Three.js.
Preparation: Technologies and Resources
Technology Stack
Required Resources
High-resolution earth texture
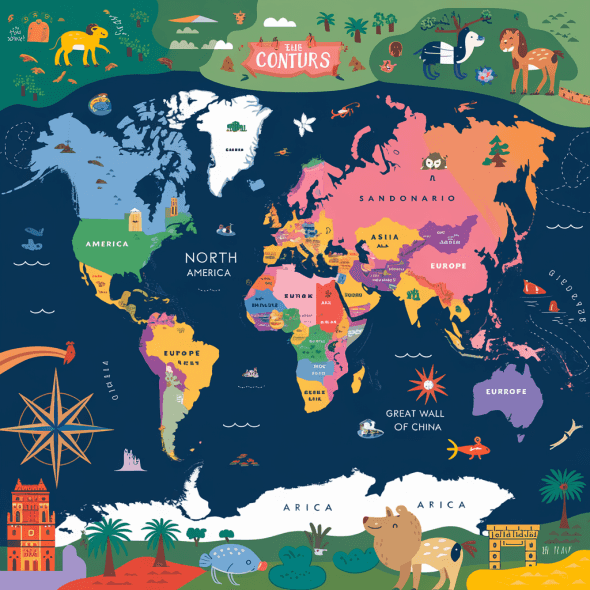
1. Project Initialization
Quick project setup with Vite:
Change the index.html
file to include the main js file:
2. Create a 3D Scene
In the main.js
file, set up the Three.js scene:
Understanding the Code: A Line-by-Line Breakdown
1. Scene Setup
This line creates the fundamental 3D container. Think of it as a digital stage where all your 3D objects will perform.
2. Camera Configuration
The camera mimics human vision:
- 75: Viewing angle (degrees)
- Aspect ratio ensures proper scaling
- Clipping planes prevent rendering unnecessary details
3. Renderer Creation
Translates 3D scene to 2D screen, handling all WebGL complexities automatically.
4. Texture and Sphere Creation
Breaks down into:
- Load texture image
- Create sphere geometry
- Apply texture as material
- Combine into a mesh
- Add to scene group
5. Interactive Elements
Captures mouse movement to enable dynamic camera positioning.
6. Animation Loop
Continuously updates:
- Camera position based on mouse
- Earth rotation
- Renders each frame
Key Optimization Techniques
- Smooth camera movement using interpolation
- Minimal rotation speeds for natural effect
- Efficient WebGL rendering
Performance Considerations
- Use lower polygon count for faster rendering
- Optimize texture sizes
- Implement lazy loading
Expansion and Improvement Possibilities
- Add cloud layer animation
- Implement country/region highlighting
- Integrate geographic data visualization
Conclusion
Three.js transforms complex 3D rendering into a straightforward, developer-friendly process. By understanding these fundamental concepts, you can create stunning interactive visualizations. Happy coding! 🚀🌍