7 Powerful JavaScript Inheritance Techniques
10 November 20243 min read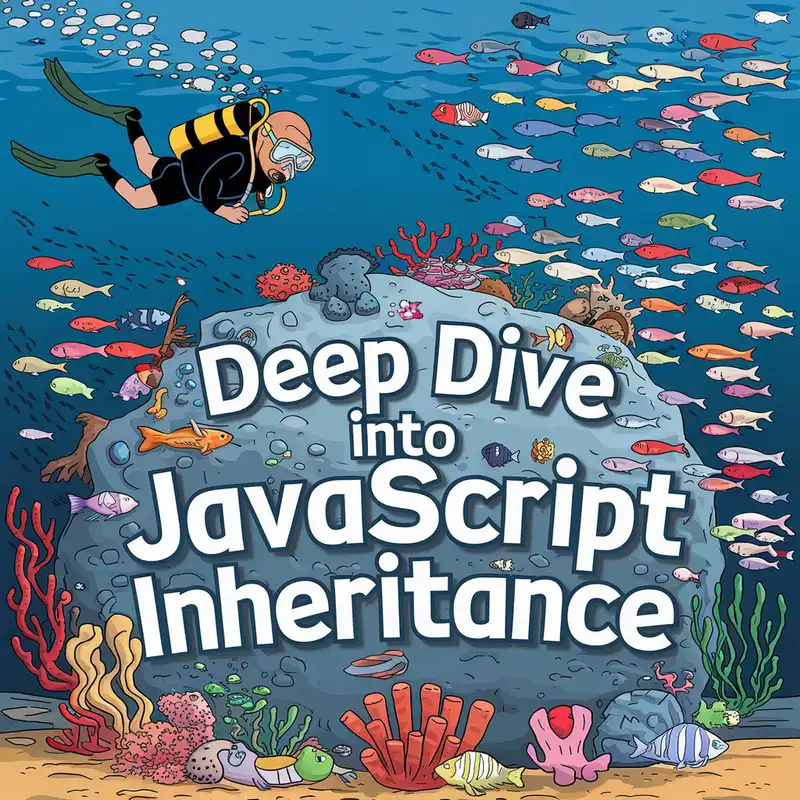
In JavaScript, inheritance is an essential way to reuse code and manage complex object relationships. With its prototype-based model, JavaScript has several inheritance patterns, each offering unique benefits. Let's explore these techniques and understand when each one is most effective.
This guide explores the seven main types of inheritance in JavaScript: from the foundational prototype chain inheritance to modern ES6 class inheritance. Each method has unique strengths and drawbacks, allowing developers to choose the best approach for various scenarios and performance needs. By understanding and implementing these inheritance models, you'll be better equipped to create structured, maintainable code in JavaScript.
1. Prototype Chain Inheritance
Prototype chain inheritance is one of the most straightforward inheritance methods. It allows an object to inherit properties and methods through a "chain" connected by prototypes.
Code Example:
1 function Animal() {2 this.species = "Mammal";3 this.habits = ["sleep", "eat"];4 }5 function Dog() {6 this.breed = "Bulldog";7 }8 Dog.prototype = new Animal();910 let d1 = new Dog();11 let d2 = new Dog();12 d1.habits.push("bark");
- Pros: Simple setup; gives subclasses access to all parent prototype methods.
- Cons: Shared reference types can lead to side effects; the parent constructor is invoked for each instance.
2. Constructor Inheritance
In this pattern, child objects inherit properties by calling the parent’s constructor directly within the child constructor.
Code Example:
1 function Animal(species) {2 this.species = species;3 this.activities = [];4 }5 function Dog(breed) {6 Animal.call(this, "Mammal");7 this.breed = breed;8 }
- Pros: Each instance has distinct properties; parameters can be passed to the parent.
- Cons: No method reuse across instances, causing potential redundancy.
3. Composite Inheritance
Composite inheritance merges prototype chain and constructor inheritance to provide unique properties to subclasses and access to parent methods.
Code Example:
1 function Animal(species) {2 this.species = species;3 this.activities = [];4 }5 Animal.prototype.getSpecies = function () {6 return this.species;7 };8 function Dog(breed) {9 Animal.call(this, "Mammal");10 this.breed = breed;11 }12 Dog.prototype = new Animal();13 Dog.prototype.constructor = Dog;
- Pros: Subclasses have their own properties and prototype methods.
- Cons: Parent constructor is called twice, increasing overhead.
4. Parasitic Inheritance
In parasitic inheritance, an object is created, modified, and returned, making the inheritance flexible but adding complexity.
Code Example:
1 function Animal() {2 this.species = "Mammal";3 this.habits = ["eat", "sleep"];4 }5 function Dog() {6 Animal.call(this);7 this.breed = "Bulldog";8 }9 Dog.prototype = Object.create(Animal.prototype);10 Dog.prototype.constructor = Dog;
- Pros: Allows prototype extensions on cloned objects.
- Cons: Less suited for large inheritance structures.
5. Prototype Inheritance
This pattern uses Object.create
to directly create an object with a specified prototype.
1 let animal = {2 species: "Mammal",3 traits: ["warm-blooded", "vertebrate"],4 };5 function createClone(obj) {6 let clone = Object.create(obj);7 clone.getTraits = function () {8 return this.traits;9 };10 return clone;11 }12 let dog = createClone(animal);
- Pros: Simple and doesn’t require constructors.
- Cons: All instances share prototype properties.
6. Parasitic Compositional Inheritance
An improved composite inheritance that avoids multiple constructor calls by copying properties with Object.create
.
Code Example:
1 function Animal(species) {2 this.species = species;3 this.traits = [];4 }5 function Dog(breed) {6 Animal.call(this, "Mammal");7 this.breed = breed;8 }9 Dog.prototype = Object.create(Animal.prototype);10 Dog.prototype.constructor = Dog;
- Pros: Solves double constructor invocation; retains composite benefits.
- Cons: Code complexity and additional encapsulation required.
7. ES6 Class Inheritance
With ES6, JavaScript introduced class
syntax, making inheritance more intuitive for developers.
Code Example:
1 class Animal {2 constructor(species) {3 this.species = species;4 }5 getSpecies() {6 return this.species;7 }8 }910 class Dog extends Animal {11 constructor(breed) {12 super("Mammal");13 this.breed = breed;14 }15 }
- Pros: Clean, readable syntax resembling traditional OOP.
- Cons: May require transpiling; slight performance difference compared to prototype-based methods.
Conclusion
Choosing the best inheritance method in JavaScript depends on your project needs. While ES6 class inheritance is popular for its readability, prototype-based patterns still play a critical role. Understanding these techniques helps you pick the best tool for flexible, efficient code in JavaScript.