How to Use AbortController to Manage Cancelable Asynchronous Tasks in JavaScript
18 March 20254 min read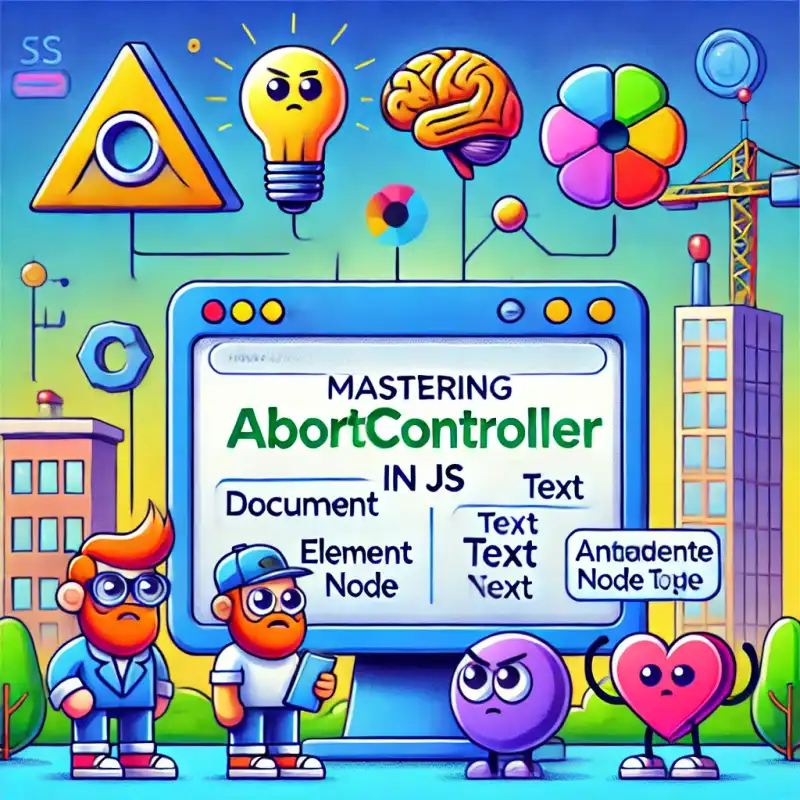
AbortController
is a powerful tool in JavaScript that allows you to cancel asynchronous operations at any time. It is particularly useful for network requests, timers, and data streaming. This article will explore how AbortController works, its use cases, and potential pitfalls.
What is AbortController?
AbortController provides a way to forcefully stop async operations in JavaScript. Some common use cases include:
- Canceling a fetch() request if it's no longer needed.
- Stopping a timer (
setTimeout()
,setInterval()
). - Aborting data streaming (
ReadableStream
).
How AbortController Works
- Create an AbortController instance:
-
js1 const abortHandler = new AbortController();
-
- Retrieve the signal from the controller:
-
js1 const abortSignal = abortHandler.signal;
-
- Pass the signal to an asynchronous operation (
fetch
,setTimeout
, etc.). - Call
.abort()
to terminate the operation when needed.
Key Methods of AbortController
AbortController.signal
Returns an AbortSignal
object that tracks when an async operation should be aborted.
1 const abortHandler = new AbortController();2 console.log(abortHandler.signal); // AbortSignal { aborted: false }
AbortController.abort()
Cancels all operations linked to the signal. After calling .abort()
, signal.aborted
becomes true
.
1 const abortHandler = new AbortController();2 abortHandler.abort();3 console.log(abortHandler.signal.aborted); // true
signal.addEventListener("abort", callback)
Executes a function when an operation is aborted.
1 const abortHandler = new AbortController();2 abortHandler.signal.addEventListener("abort", () =>3 console.log("Operation aborted")4 );56 setTimeout(() => abortHandler.abort(), 2000);
Canceling fetch()
Requests
Without AbortController (Not Recommended)
1 async function loadData() {2 const response = await fetch("https://api.example.com/data");3 const result = await response.json();4 console.log(result);5 }67 loadData();8 setTimeout(() => console.log("What if the request is no longer needed?"), 1000);
This request cannot be stopped, consuming unnecessary resources.
With AbortController (Recommended)
1 const abortHandler = new AbortController();2 const abortSignal = abortHandler.signal;34 async function loadData() {5 try {6 const response = await fetch("https://api.example.com/data", {7 signal: abortSignal,8 });9 const result = await response.json();10 console.log(result);11 } catch (err) {12 if (err.name === "AbortError") {13 console.log("Request was canceled!");14 } else {15 console.error("Error:", err);16 }17 }18 }1920 loadData();21 setTimeout(() => abortHandler.abort(), 1000);
This ensures that if a request is no longer needed, resources are freed up immediately.
Additional Example: Canceling Multiple Fetch Requests
1 const fetchControllers = new Map();23 async function fetchWithCancel(url, requestId) {4 if (fetchControllers.has(requestId)) {5 fetchControllers.get(requestId).abort();6 }78 const newAbortHandler = new AbortController();9 fetchControllers.set(requestId, newAbortHandler);1011 try {12 const response = await fetch(url, { signal: newAbortHandler.signal });13 const data = await response.json();14 console.log("Fetched Data:", data);15 } catch (err) {16 if (err.name === "AbortError") {17 console.log(`Request ${requestId} was canceled`);18 } else {19 console.error("Error:", err);20 }21 }22 }2324 fetchWithCancel("https://api.example.com/data", "request1");25 setTimeout(26 () => fetchWithCancel("https://api.example.com/data", "request1"),27 50028 );
Use Cases for AbortController
1. Canceling Search Requests in Input Fields
When users type in a search box, multiple API calls can be triggered. We can cancel previous calls to save resources.
1 let searchAbortHandler;23 async function search(query) {4 if (searchAbortHandler) searchAbortHandler.abort(); // Cancel previous request56 searchAbortHandler = new AbortController();7 const abortSignal = searchAbortHandler.signal;89 try {10 const response = await fetch(`https://api.example.com/search?q=${query}`, {11 signal: abortSignal,12 });13 const result = await response.json();14 console.log("Results:", result);15 } catch (err) {16 if (err.name === "AbortError") {17 console.log("Previous request canceled");18 } else {19 console.error("Error:", err);20 }21 }22 }2324 document.querySelector("#search").addEventListener("input", (e) => {25 search(e.target.value);26 });
2. Stopping Timers and Background Tasks
AbortController can manage setTimeout()
and cancel tasks before execution.
1 const timerAbortHandler = new AbortController();2 const timerAbortSignal = timerAbortHandler.signal;34 function executeDelayedTask() {5 if (timerAbortSignal.aborted) {6 console.log("Timer canceled");7 return;8 }910 setTimeout(() => {11 if (!timerAbortSignal.aborted) {12 console.log("Task executed");13 }14 }, 5000);15 }1617 setTimeout(() => timerAbortHandler.abort(), 2000);18 executeDelayedTask();
3. Canceling File Downloads (Streaming Data)
1 const downloadAbortHandler = new AbortController();2 const downloadAbortSignal = downloadAbortHandler.signal;34 async function downloadLargeFile() {5 try {6 const response = await fetch("https://example.com/largefile.zip", {7 signal: downloadAbortSignal,8 });9 const reader = response.body.getReader();10 let receivedBytes = 0;11 let chunks = [];1213 while (true) {14 const { done, value } = await reader.read();15 if (done) break;16 chunks.push(value);17 receivedBytes += value.length;18 console.log(`Downloaded ${receivedBytes} bytes`);19 }20 } catch (err) {21 if (err.name === "AbortError") {22 console.log("Download canceled");23 } else {24 console.error("Error:", err);25 }26 }27 }2829 document30 .querySelector("#startDownload")31 .addEventListener("click", downloadLargeFile);32 document33 .querySelector("#cancelDownload")34 .addEventListener("click", () => downloadAbortHandler.abort());
Conclusion
AbortController is a valuable tool for managing asynchronous operations efficiently in JavaScript. By integrating it into your code, you can improve performance, reduce resource waste, and enhance user experience.
What are your experiences with AbortController? Share your thoughts in the comments!