Understanding JavaScript Memory Management: Deep Dive into Garbage Collection Techniques
3 April 20255 min read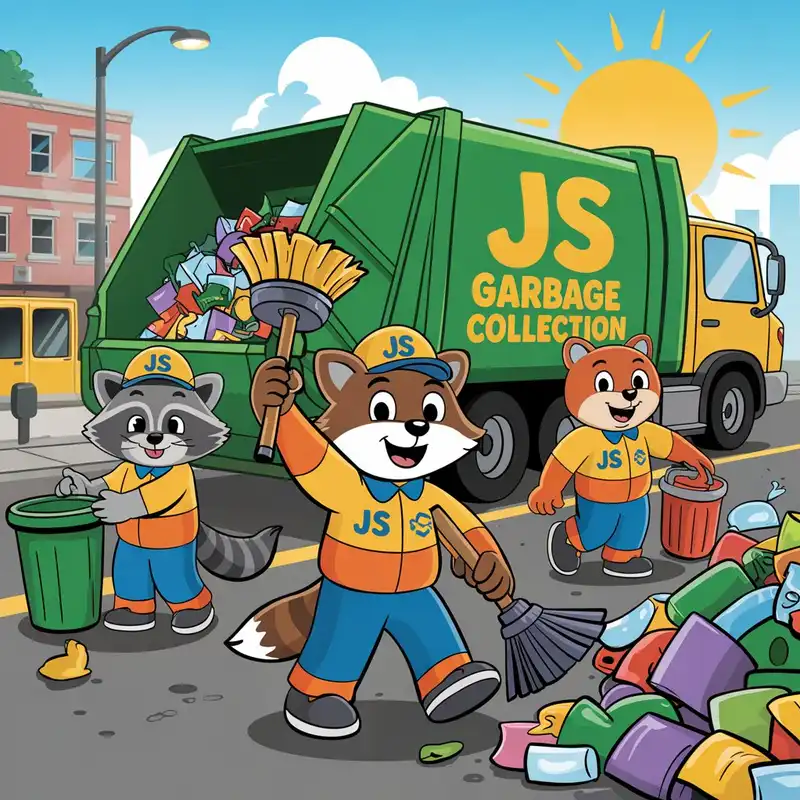
In JavaScript, memory management is vital for both performance and stability. The garbage collection (GC) mechanism handles automatic memory reclamation, but there are several algorithms employed to manage this efficiently. Below is an in-depth exploration of the three classic GC algorithms:
1. In-depth Analysis of Three Garbage Collection Algorithms
Mark-and-Sweep Algorithm
Core Principle: Identify surviving objects through reachability analysis. Process:
- Marking: Start from GC roots, such as global objects and active function call stacks.
- Tracking: Perform depth-first traversal to find all reachable objects.
- Cleanup: Reclaim memory from unmarked objects.
1 function markAndSweep() {2 // Mark phase3 let worklist = [...roots];4 while (worklist.length > 0) {5 const obj = worklist.pop();6 if (!obj.marked) {7 obj.marked = true;8 worklist.push(...obj.references);9 }10 }1112 // Sweep phase13 for (const obj of heap) {14 if (!obj.marked) {15 freeMemory(obj);16 } else {17 obj.marked = false; // Reset marking18 }19 }20 }
Advantages:
- Solves the circular reference problem.
- Efficient with large-scale memory management.
- High memory utilization.
Disadvantages:
- Memory fragmentation (though this can be optimized with mark-defragmentation).
- Full heap scan can cause Stop-the-World (STW) pauses.
Reference Counting
Core Principle: Track object lifecycle using a reference counter.
1 class RefCountedObject {2 constructor() {3 this.count = 1; // Initial reference count4 this.references = new Set();5 }67 addRef() {8 this.count++;9 }1011 release() {12 if (--this.count === 0) {13 this._destroy();14 }15 }1617 _destroy() {18 this.references.forEach(obj => obj.release());19 freeMemory(this);20 }21 }
Pitfall:
- Circular Reference Issue: Objects that reference each other can never reach zero count, causing a memory leak.
1 function createCycle() {2 let objA = new RefCountedObject();3 let objB = new RefCountedObject();45 objA.ref = objB; // objB.count = 26 objB.ref = objA; // objA.count = 27 }89 createCycle();10 // After execution: objA.count = 1, objB.count = 1 → Memory leak
Modern Usage:
- Used in specialized cases (e.g., COM components).
- Forms the basis for APIs like
WeakRef
. read also Master JavaScript WeakRef & FinalizationRegistry - Often combined with mark-and-sweep for better efficiency.
Generational Garbage Collection
Core Principle: The Weak Generation Hypothesis suggests younger objects tend to die faster. V8 Specific Implementation: Objects that survive several GC cycles in the "new generation" are promoted to the "old generation."
1 // Example: New Space Memory Layout (Semispace)2 class NewSpace {3 constructor() {4 this.fromSpace = new ArrayBuffer(4 * 1024 * 1024); // 4MB5 this.toSpace = new ArrayBuffer(4 * 1024 * 1024);6 this.allocPtr = 0;7 }89 allocate(size) {10 if (this.allocPtr + size > this.fromSpace.byteLength) {11 this.doScavenge();12 }13 const ptr = this.allocPtr;14 this.allocPtr += size;15 return ptr;16 }1718 doScavenge() {19 // Cheney's Algorithm to copy live objects20 let scanPtr = 0;21 this.allocPtr = 0;2223 while (scanPtr < this.allocPtr) {24 const obj = this.toSpace[scanPtr];25 for (const ref of obj.references) {26 if (ref.isInFromSpace()) {27 copyToNewSpace(ref);28 }29 }30 scanPtr += obj.size;31 }32 [this.fromSpace, this.toSpace] = [this.toSpace, this.fromSpace];33 }34 }
Comparison of generational strategies:
Generation | Proportion | GC Frequency | Algorithm | Optimization Target |
---|---|---|---|---|
Young Generation | 5% | High frequency | Scavenge | Throughput |
Old Generation | 95% | Low frequency | Mark-sweep/collection | Latency control |
2. Evolution of Modern GC Algorithms
New Generation Scavenge Algorithm
The Cheney algorithm facilitates fast recycling by alternating between "From" and "To" spaces:
1 function scavenge() {2 let from = currentNewSpace;3 let to = newNewSpace;45 for (let obj of reachableObjects) {6 copyObject(obj, to);7 forwardPointer(obj, to);8 }910 swapSpaces(from, to);11 }
Old Generation Mark-Sweep Optimization
V8 optimizes the old generation using a three-color marking method combined with incremental marking to avoid long STW pauses:
- White: Unvisited objects
- Gray: Objects being accessed
- Black: Objects already visited
1 function incrementalMarking() {2 let worklist = getGreyObjects();34 while (worklist.length > 0 && !shouldYield()) {5 const obj = worklist.pop();6 markObject(obj);7 for (const ref of getReferences(obj)) {8 if (!isMarked(ref)) {9 markGrey(ref);10 worklist.push(ref);11 }12 }13 }1415 if (worklist.length > 0) {16 requestIdleCallback(incrementalMarking);17 }18 }
3. Modern Forms of Memory Leaks
Closure Trap
1 function createClosureLeak() {2 const hugeData = new Array(1e6).fill('*');3 return function() {4 // Closure inadvertently holds onto hugeData5 console.log('Closure executed');6 };7 }89 let leakedClosure = createClosureLeak();
Reference Relics in Modern Frameworks (React Example)
1 function Component() {2 const [data, setData] = useState(null);34 useEffect(() => {5 const timer = setInterval(() => {6 fetchData().then(result => {7 setData(result); // May hold old references if missing dependencies8 });9 }, 1000);1011 return () => clearInterval(timer);12 }, []); // Missing dependency could cause memory leaks13 }
4. GC Performance Optimization Strategies
Object Pooling
By reusing memory through object pools, you can minimize the need for frequent garbage collection.
1 class VectorPool {2 constructor() {3 this.pool = [];4 }56 create(x, y) {7 return this.pool.pop() || { x, y };8 }910 release(vec) {11 vec.x = null;12 vec.y = null;13 this.pool.push(vec);14 }15 }1617 // Usage example18 const pool = new VectorPool();19 const v1 = pool.create(10, 20);20 // After usage21 pool.release(v1);
Optimizing Memory Access Patterns
Improving memory access patterns can lead to better cache utilization, reducing the performance impact of GC.
1 // Inefficient access pattern2 function processMatrix(matrix) {3 for (let col = 0; col < 1000; col++) {4 for (let row = 0; row < 1000; row++) {5 process(matrix[row][col]); // Breaks memory locality6 }7 }8 }910 // Optimized access pattern11 function optimizedProcess(matrix) {12 for (let row = 0; row < 1000; row++) {13 const rowData = matrix[row];14 for (let col = 0; col < 1000; col++) {15 process(rowData[col]); // Sequential access16 }17 }18 }
Conclusion
Garbage collection in JavaScript plays a critical role in optimizing both memory and performance. While automatic GC can help prevent memory leaks, understanding its mechanisms and strategically optimizing your code can lead to significant performance gains. The balance between automatic and manual memory management is key to developing high-performance web applications.