The Complete Guide to JavaScript Timer Management: Mastering clearTimeout and clearInterval
17 March 20252 min read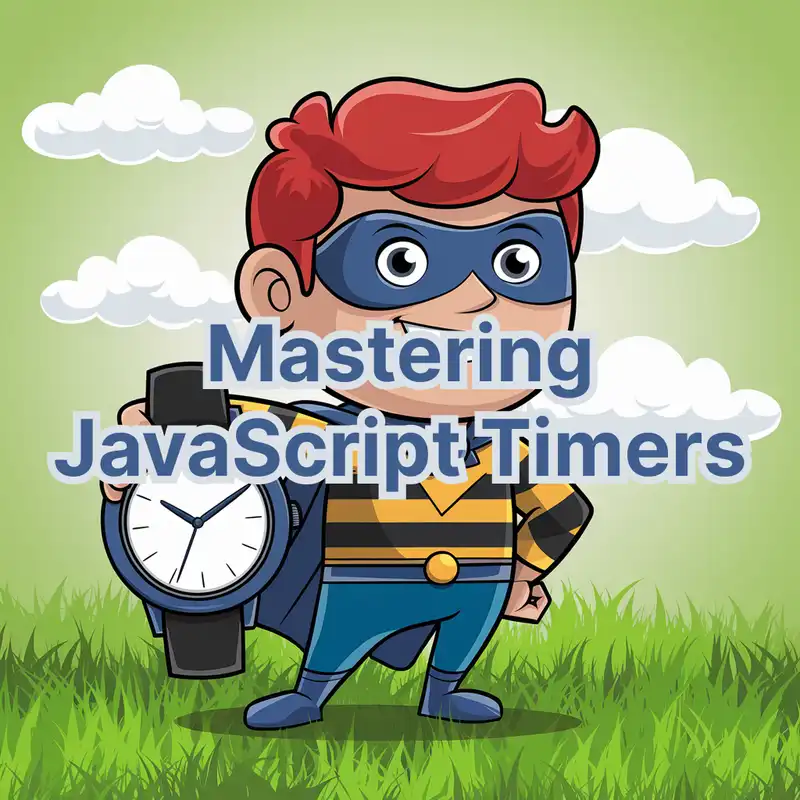
In JavaScript, timers help execute code after a delay or at regular intervals. The most common timers are setTimeout
and setInterval
. However, improperly managed timers can lead to memory leaks or unintended behavior. This guide covers different methods to clear timers efficiently.
1. Clearing a setTimeout
Timer
setTimeout
executes code once after a specified delay and returns a timer ID. Use clearTimeout
to cancel it before execution.
1 const timerId = setTimeout(() => {2 console.log("Timer executed");3 }, 1000);45 clearTimeout(timerId); // Cancels the timeout
2. Clearing a setInterval Timer
setInterval
repeatedly executes code at specified intervals. Use clearInterval
to stop it.
1 const intervalId = setInterval(() => {2 console.log("Interval executed");3 }, 1000);45 clearInterval(intervalId); // Stops the interval
3. Clearing Multiple Timers
Store multiple timer IDs in an array and iterate over them to clear all timers.
1 const timerIds = [];2 timerIds.push(setTimeout(() => console.log("Timer 1"), 1000));3 timerIds.push(setTimeout(() => console.log("Timer 2"), 2000));45 timerIds.forEach(clearTimeout); // Clears all timeouts
4. Clearing Timers on Component Unmount (React Example)
In React, ensure timers are cleared when a component unmounts to prevent memory leaks.
1 import React, { useEffect } from "react";23 function MyComponent() {4 useEffect(() => {5 const timerId = setTimeout(() => {6 console.log("Timer executed");7 }, 1000);89 return () => clearTimeout(timerId); // Cleanup on unmount10 }, []);1112 return <div>Component Content</div>;13 }
5. Using a Promise to Manage Timers
Encapsulate timers in a Promise for better control.
1 function delay(ms) {2 let timerId;3 const promise = new Promise((resolve) => {4 timerId = setTimeout(resolve, ms);5 });6 return { promise, timerId };7 }89 const { promise, timerId } = delay(1000);10 promise.then(() => console.log("Timer executed"));1112 clearTimeout(timerId); // Cancel if needed
6. Using AbortController
to Cancel a Timer
AbortController
provides a way to cancel timers programmatically.
1 function delay(ms, signal) {2 return new Promise((resolve, reject) => {3 const timerId = setTimeout(resolve, ms);4 signal.addEventListener("abort", () => {5 clearTimeout(timerId);6 reject(new Error("Timer aborted"));7 });8 });9 }1011 const controller = new AbortController();12 delay(1000, controller.signal)13 .then(() => console.log("Timer executed"))14 .catch((err) => console.log(err.message));1516 controller.abort(); // Cancels the timer
7. Clearing All Active Timers
For environments where you need to clear all running timers dynamically:
1 function clearAllTimers() {2 let id = setTimeout(() => {}, 0);3 while (id >= 0) {4 clearTimeout(id);5 id--;6 }7 }89 clearAllTimers(); // Clears all active timeouts
Summary
Clearing timers is essential for efficient resource management. Use clearTimeout
to cancel a delayed execution and clearInterval
to stop repeated executions. When dealing with multiple timers, store their IDs in an array and clear them in a loop. In React, ensure timers are cleaned up when components unmount to prevent memory leaks. Using Promises and AbortController
provides better control over timer execution. If necessary, implement a function to clear all active timers dynamically. Proper timer management helps improve performance and prevents unintended behavior in JavaScript applications.