Pure CSS: Infinite Horizontal Scrolling Logo Display
10 November 20244 min read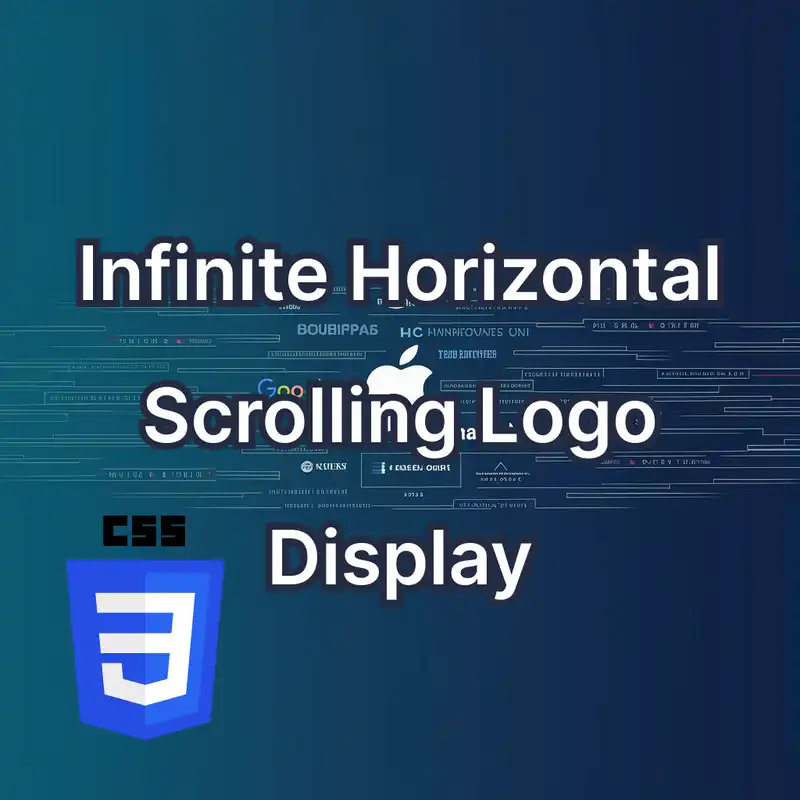
Creating an infinite horizontal logo scroll effect with pure CSS is a simple yet powerful way to add dynamic movement to a website. This tutorial will walk you through building an infinite scroll effect with added hover effects that allow the user to pause scrolling and scale each logo slightly when hovered.
Step 1: HTML Structure
To start, we’ll create an HTML structure with a container that holds all the logos. To ensure a smooth infinite scroll effect, each logo will be duplicated.
1 <!DOCTYPE html>2 <html lang="en">3 <head>4 <meta charset="UTF-8" />5 <meta name="viewport" content="width=device-width, initial-scale=1.0" />6 <title>Build an Infinite Horizontal Scrolling Logo Display</title>7 <link rel="stylesheet" href="style.css" />8 </head>9 <body>10 <div class="logo-container">11 <div class="logo-scroll">12 <div class="logo-scroll__wrapper">13 <div class="logo-item"></div>14 <div class="logo-item"></div>15 <div class="logo-item"></div>16 <div class="logo-item"></div>17 <div class="logo-item"></div>18 <div class="logo-item"></div>19 <div class="logo-item"></div>20 <div class="logo-item"></div>21 </div>22 <div class="logo-scroll__wrapper">23 <div class="logo-item"></div>24 <div class="logo-item"></div>25 <div class="logo-item"></div>26 <div class="logo-item"></div>27 <div class="logo-item"></div>28 <div class="logo-item"></div>29 <div class="logo-item"></div>30 <div class="logo-item"></div>31 </div>32 </div>33 </div>34 </body>35 </html>
This HTML structure sets up the necessary elements to create an infinite horizontal scrolling logo display. The logos will be placed inside logo-item
divs, which are grouped into logo-scroll__wrapper
divs. These wrappers are animated via CSS to scroll infinitely.
This HTML is structured to work seamlessly with the provided CSS for smooth, infinite scrolling, and scalable logo elements.
Step 2: CSS Styling and Animation
Now we’ll add the CSS styles to control the scrolling effect, add a hover-pause, and scale the logos slightly on hover.
1 /* Container to limit the scroll width */2 .logo-container {3 /* Setting custom properties for logo dimensions, spacing, and animation duration */4 --logo-width: 200px; /* Width of each logo */5 --logo-height: 100px; /* Height of each logo */6 --gap: calc(7 var(--logo-width) / 148 ); /* Space between logos, calculated based on logo width */9 --duration: 60s; /* Duration of the scroll animation */10 --scroll-start: 0; /* Start position of scroll animation */11 --scroll-end: calc(-100% - var(--gap)); /* End position of scroll animation */1213 display: flex; /* Aligns children in a row */14 flex-direction: column; /* Stacks content vertically */15 gap: var(--gap); /* Adds space between child elements */16 margin: auto; /* Centers container horizontally */17 max-width: 100vw; /* Limits width to the viewport */18 }1920 /* Scrolling area */21 .logo-scroll {22 display: flex; /* Aligns logos in a horizontal row */23 overflow: hidden; /* Hides overflow to create infinite scroll effect */24 user-select: none; /* Disables text selection */25 gap: var(--gap); /* Space between logos */26 mask-image: linear-gradient(27 to right,28 hsl(0 0% 0% / 0),29 hsl(0 0% 0% / 1) 30%,30 hsl(0 0% 0% / 1) 70%,31 hsl(0 0% 0% / 0)32 ); /* Adds a gradient mask to fade edges */33 }3435 .logo-scroll__wrapper {36 flex-shrink: 0; /* Prevents wrapper from shrinking */37 display: flex; /* Aligns logos horizontally */38 align-items: center; /* Centers logos vertically */39 justify-content: space-around; /* Distributes logos evenly */40 gap: var(--gap); /* Adds spacing between logos */41 min-width: 100%; /* Wrapper width covers full viewport */42 animation: scroll var(--duration) linear infinite; /* Infinite scrolling animation */43 }4445 .logo-scroll__wrapper:nth-child(even) {46 margin-left: calc(47 var(--logo-width) / -248 ); /* Offsets even wrappers for smooth scroll overlap */49 }5051 .logo-scroll__wrapper:hover {52 animation-play-state: paused; /* Pauses animation when wrapper is hovered */53 }5455 /* Logo styling */56 .logo-item {57 width: var(--logo-width); /* Sets width of each logo */58 height: var(--logo-height); /* Sets height of each logo */59 /* transition: transform 0.5s; Smooth scaling effect */60 background-color: blue; /* Background color for logos */61 border-radius: 4px; /* Rounds the logo corners */62 }6364 .logo-scroll .logo-item:hover {65 transform: scale(1.05); /* Slightly enlarges logo when hovered */66 }6768 /* Infinite scroll animation */69 @keyframes scroll {70 from {71 transform: translateX(var(--scroll-start)); /* Animation start position */72 }73 to {74 transform: translateX(var(--scroll-end)); /* Animation end position */75 }76 }
Summary of Functionality:
- Container and Scroll Properties: The
.logo-container
sets up the layout, .logo-scroll
manages the scroll area, and.logo-scroll__wrapper
animates the scrolling. - Hover Effects: Hovering over
.logo-scroll__wrapper
pauses the scroll animation, and hovering over.logo-item
scales the logos slightly for interaction. - Infinite Scroll Animation: The
@keyframes scroll
moves the entire.logo-scroll__wrapper
horizontally from the start to end position, creating a seamless loop.
This setup is fully responsive and adaptable; adjust sizes, timings, and spacing as needed for your layout.
These combined elements create a smooth, interactive, infinite-scrolling logo display with CSS-only animations.
Tips for Optimization
- Scroll Speed: Adjust the 60s duration for faster or slower scrolling.
- Hover Effect: Try different scaling percentages or easing effects to customize the hover appearance.
- Responsive Adjustments: You can set logo sizes with relative units like vw or em for responsive behavior.
Result
This approach is purely CSS-based, easy to implement, and a great way to add interactive design elements to a webpage without JavaScript. Adjust the styling as needed to create a smooth, visually appealing, and responsive scrolling logo display.