Elegant Long Press Implementation Using CSS Animation
Add to your RSS feed5 January 20252 min read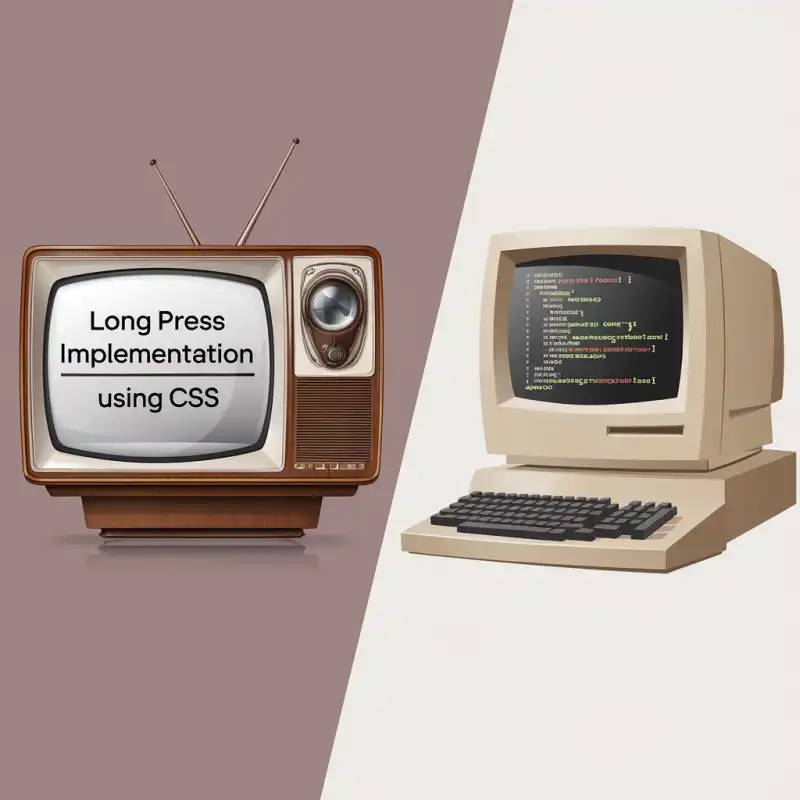
Table of Contents
Handling different user interactions, such as short and long presses, is a common task in web development. This article introduces a sleek way to implement handlers for both types of interactions.
Why Use Long Press?
Long Press is triggered when a user holds down a button or screen for a specific duration. It’s useful for invoking additional actions like context menus, tooltips, or advanced features that aren’t triggered by a regular click.
Conventional JavaScript Approach
A traditional implementation might look like this:
1 <!-- HTML -->2 <button id="btn">Click / Long Press</button>34 <!-- JavaScript -->5 const btn = document.getElementById('btn');6 let timerId, longPressed;78 btn.onmousedown = () => {9 longPressed = false;10 timerId = setTimeout(() => {11 longPressed = true;12 console.log('Long Press Handler');13 }, 200);14 };1516 btn.onclick = () => {17 if (!longPressed) console.log('Click Handler');18 clearTimeout(timerId);19 };2021 btn.onmouseleave = () => clearTimeout(timerId);
This approach, while functional, involves timers, global variables, and additional logic to handle edge cases (e.g., releasing the button outside its boundaries).
Universal CSS-Based Solution
By leveraging CSS animations, you can simplify this process:
1 <!-- HTML -->2 <button id="btn">Click / Long Press</button>34 <!-- CSS -->5 #btn {6 transition: background-color 1s 200ms;7 }89 #btn:active {10 animation-name: interruptClick;11 animation-delay: 200ms;12 animation-fill-mode: forwards;13 background-color: PaleTurquoise;14 }1516 #btn:not(:hover) {17 animation-play-state: paused;18 }1920 @keyframes interruptClick {21 to {22 pointer-events: none;23 }24 }2526 /* JavaScript */27 const btn = document.getElementById('btn');28 btn.onclick = () => console.log('Click Handler');29 btn.onanimationend = () => console.log('Long Press Animation End');
How It Works
- CSS Animation for Long Press
:active
: Triggers only while the button is held down.animation-delay
: Sets a delay (e.g., 200ms) to define a long press.pointer-events
: none: Prevents a click event after the animation ends.:not(:hover)
: Stops the animation if the button is released outside its bounds.
- JavaScript Listeners
onclick
: Handles short presses.onanimationend
: Handles long presses when the animation completes.
Demo on CodePen
Benefits of the CSS Approach
- Simplicity: Eliminates the need for timers or complex JavaScript logic.
- Performance: Reduces JavaScript computations by offloading visual effects to CSS.
- Readability: Keeps the code clean and easy to maintain.
- Cross-Browser Compatibility: Works well with minimal browser-specific adjustments.
Conclusion
This method showcases the powerful synergy between CSS and JavaScript, enabling clean and efficient code for user interaction. Ensure thorough testing across devices and browsers before deploying to production.