Using useActionState for Advanced React State Management
Add to your RSS feed9 January 20255 min read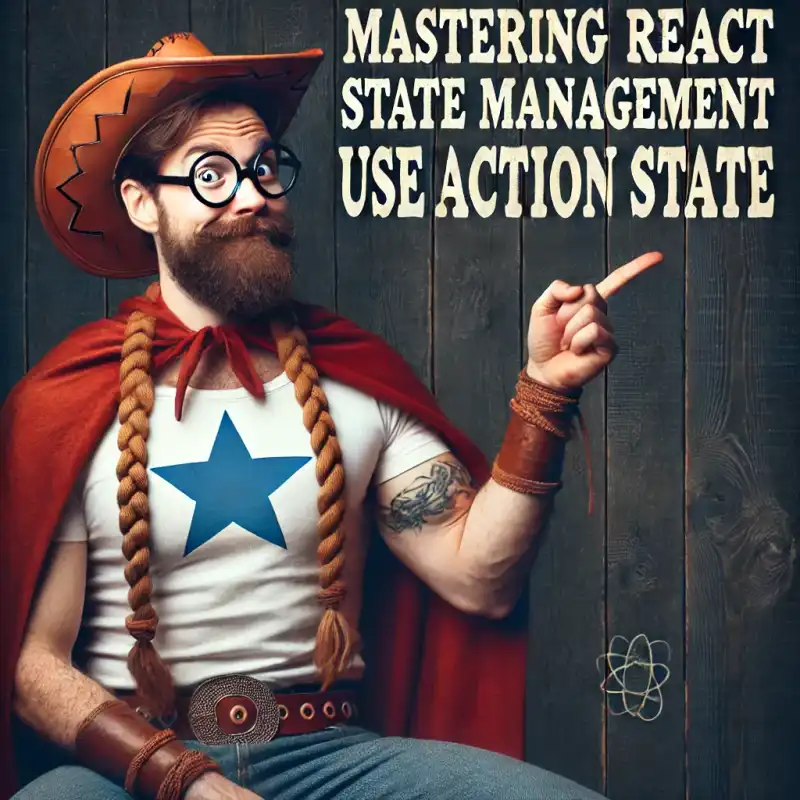
Table of Contents
State management is an integral part of React development, and choosing the right approach can often dictate the maintainability and readability of your code. While useState
and useReducer
serve as foundational tools for managing state in React, they can fall short when dealing with scenarios that involve asynchronous actions or consolidated state updates. Enter useActionState
, a relatively new addition to the React ecosystem, designed to simplify state management by linking state transitions directly to actions. This article delves deep into the capabilities of useActionState
, offering insights, use cases, and examples to help you harness its power effectively.
The Evolution of React State Management
Before diving into useActionState
, let’s take a moment to understand its origins. Historically, React developers have relied on useState
for managing local state and useReducer
for more complex state transitions. While these hooks are powerful, they require verbose code when dealing with multiple interdependent states or actions.
For example, consider managing form submission states, where you might track:
- Whether a form is being submitted.
- The success or failure of the submission.
- Any error messages or feedback for the user.
Using useState
, this often translates to multiple state variables:
1 const [isSubmitting, setIsSubmitting] = useState(false);2 const [successMessage, setSuccessMessage] = useState('');3 const [errorMessage, setErrorMessage] = useState('');
This approach works but becomes unwieldy as the number of state variables grows. Moreover, it’s not inherently tied to the action that triggers these state changes. This is where useActionState
shines.
Introducing useActionState
useActionState
is a hook that bridges the gap between actions and state transitions. It allows you to define actions that update state in a single, cohesive function, streamlining your code and improving its readability. The hook returns three values:
- Current State: The current state value, which can be of any type.
- Action Function: A function that triggers the action and updates the state.
- isPending: A boolean flag indicating whether the action is in progress.
Here’s the basic syntax:
1 const [currentState, actionFunction, isPending] = useActionState(fn, initialState, permalink?);
Parameters
fn
: The primary function that executes the action and returns the updated state.initialState
: The initial value of the state.permalink
(optional): A unique URL for fallback behavior, useful for server-side rendering.
Why Choose useActionState?
The main advantage of useActionState
lies in its simplicity and versatility. It consolidates state updates, action triggers, and loading states into a single hook, making your code cleaner and easier to maintain. Here are some key benefits:
- Reduced Boilerplate: Eliminate the need for multiple
useState
hooks to manage related states. - Built-in Loading State: Automatically track the progress of asynchronous actions with the
isPending
flag. - Action-Oriented Design: Tie state transitions directly to specific actions, improving clarity.
- Server-Side Compatibility: Integrate seamlessly with server-side frameworks like Next.js.
Practical Examples
To better understand useActionState
, let’s explore some real-world examples.
Example 1: Counter with Action State
A simple counter application demonstrates how useActionState
can simplify state management:
1 const incrementCounter = async (prevCount) => prevCount + 1;23 function Counter() {4 const [count, increment, isPending] = useActionState(incrementCounter, 0);56 return (7 <div>8 <p>Count: {count}</p>9 <button onClick={increment} disabled={isPending}>10 {isPending ? 'Processing...' : 'Increment'}11 </button>12 </div>13 );14 }
In this example, the incrementCounter
function updates the count. The isPending
flag prevents multiple increments while the action is in progress.
Example 2: File Upload Form
Let’s move on to a more complex use case—managing file uploads with feedback for the user:
1 async function uploadFile(prevState, formData) {2 try {3 await new Promise((resolve) => setTimeout(resolve, 2000));4 return { success: true, message: 'File uploaded successfully!' };5 } catch {6 return { success: false, message: 'Upload failed.' };7 }8 }910 function UploadForm() {11 const [uploadStatus, uploadAction, isUploading] = useActionState(uploadFile, null);1213 return (14 <form>15 <input type="file" name="file" />16 <button formAction={uploadAction} disabled={isUploading}>17 {isUploading ? 'Uploading...' : 'Upload'}18 </button>1920 {uploadStatus && (21 <p className={uploadStatus.success ? 'success' : 'error'}>22 {uploadStatus.message}23 </p>24 )}25 </form>26 );27 }
Here, the uploadFile
function handles the file upload logic. The state (uploadStatus
) contains information about the success or failure of the upload.
Example 3: Fetching Data from a Server
Fetching data from a server is another common use case. With useActionState
, it becomes straightforward:
1 import { getDataFromServer } from './actions.server.js'; // "use server"23 function FetchServerData() {4 const [data, fetchData, isPending] = useActionState(async () => {5 return await getDataFromServer();6 }, null);78 return (9 <div>10 <button onClick={fetchData} disabled={isPending}>11 {isPending ? 'Fetching...' : 'Fetch Data'}12 </button>13 {data && <p>Server Response: {data}</p>}14 </div>15 );16 }1718 // actions.server.js19 "use server";2021 export async function getDataFromServer() {22 // Simulate a server request23 return 'Hello from the server!';24 }
What Happens Here:
- The
fetchData
action triggers a server request. - The
isPending
flag provides immediate feedback during the request. - The
data
state holds the server response.
Best Practices with useActionState
To maximize the benefits of useActionState
, consider these best practices:
- Define Meaningful Initial States: Ensure the initial state reflects the expected structure of your data.
- Handle Errors Gracefully: Return structured results with
success
anderror
fields for consistent error handling. - Use isPending Wisely: Leverage the built-in loading state to enhance user experience.
- Focus on Single-Action Workflows: Use
useActionState
for actions like form submissions, file uploads, or server requests. - Combine with Server Components: Take advantage of server-side rendering and server actions for better performance.
Limitations and Considerations
While useActionState
is powerful, it’s not a one-size-fits-all solution. For complex forms with numerous fields or intricate state transitions, useReducer
or controlled components may still be a better choice. useActionState
excels in scenarios where a single action drives the state change.
Conclusion
useActionState
is a significant addition to React’s state management toolbox. By consolidating state updates and action logic, it simplifies code and enhances clarity. Whether you’re managing server interactions, handling user input, or tracking asynchronous processes, useActionState
provides a clean and efficient approach.
Try incorporating useActionState
into your next project to experience its advantages firsthand. With its action-oriented design and built-in features, it’s a valuable tool for modern React development.