Boost React Performance: Tips for Faster Applications
19 January 20254 min read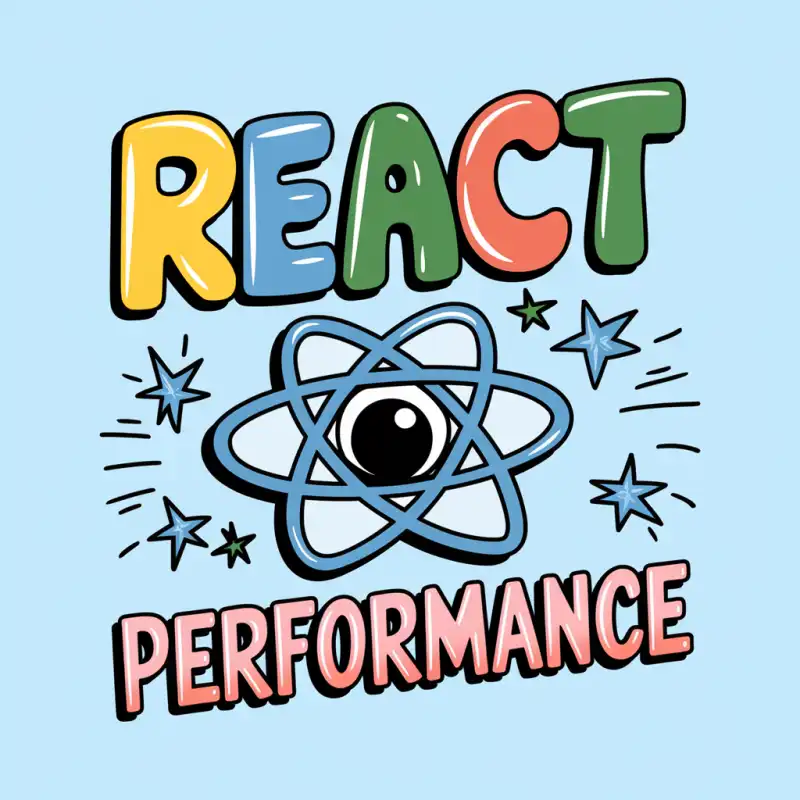
React is a powerful library for building user interfaces, but optimizing performance can be challenging, especially as applications grow in complexity. This comprehensive guide explores best practices to optimize React applications, including rendering mechanisms, virtualization, lazy loading, build enhancements, and server-side rendering (SSR). Let’s delve into each topic with detailed explanations and code examples.
Performance optimization is crucial for creating responsive and efficient React applications. This comprehensive guide covers essential techniques and best practices to enhance both your app's speed and development workflow.
1. React Rendering Mechanism Optimization
React uses a virtual DOM to efficiently update the user interface. However, unnecessary re-renders can impact performance. Here’s how to optimize rendering:
1.1 Using React.memo
and PureComponent
React.memo
: Prevents re-renders for function components when props remain unchanged.PureComponent
: Optimizes class components by shallowly comparing props and state.
Example: React.memo
1 const ChildComponent = React.memo(({ value }) => {2 console.log('ChildComponent rendered');3 return <div>{value}</div>;4 });56 const ParentComponent = () => {7 const [count, setCount] = React.useState(0);8 return (9 <div>10 <button onClick={() => setCount(count + 1)}>Increase Count</button>11 <ChildComponent value={count} />12 </div>13 );14 };
In this example, ChildComponent
only re-renders when its value
prop changes.
1.2 Using useMemo and useCallback
useMemo
: Caches expensive computations.useCallback
: Memoizes function references to avoid unnecessary re-creation.
Example: useMemo
1 const ExpensiveCalculation = ({ items }) => {2 const total = React.useMemo(() => {3 return items.reduce((sum, item) => sum + item.value, 0);4 }, [items]);56 return <div>Total: {total}</div>;7 };
Example: useCallback
1 const IncrementButton = () => {2 const [count, setCount] = React.useState(0);34 const increment = React.useCallback(() => {5 setCount((prev) => prev + 1);6 }, []);78 return <button onClick={increment}>Increment</button>;9 };
2. Virtualization for Large Data Sets
Rendering large lists or tables can degrade performance. Virtualization ensures only visible elements are rendered.
2.1 Using react-window
Install the library:
Example: Virtualized List
1 import { FixedSizeList as List } from 'react-window';23 const Row = ({ index, style }) => (4 <div style={style}>Item {index}</div>5 );67 const VirtualizedList = () => (8 <List9 height={400}10 itemCount={1000}11 itemSize={35}12 width={300}13 >14 {Row}15 </List>16 );
This renders only items visible within the viewport, reducing DOM nodes.
3. Lazy Loading and On-Demand Loading
3.1 Lazy Loading with React.lazy and Suspense
Lazy loading improves load times by deferring component loading until needed.
Example: Component Lazy Loading
1 const LazyComponent = React.lazy(() => import('./LazyComponent'));23 const App = () => (4 <Suspense fallback={<div>Loading...</div>}>5 <LazyComponent />6 </Suspense>7 );
3.2 Route-Based Lazy Loading with React Router
1 import React, { Suspense } from 'react';2 import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';34 const HomePage = React.lazy(() => import('./HomePage'));5 const AboutPage = React.lazy(() => import('./AboutPage'));67 const App = () => (8 <Router>9 <Suspense fallback={<div>Loading...</div>}>10 <Switch>11 <Route path="/" exact component={HomePage} />12 <Route path="/about" component={AboutPage} />13 </Switch>14 </Suspense>15 </Router>16 );
4. Build Optimization Techniques
4.1 Enable React Production Mode
Ensure production builds by running:
4.2 Code Splitting with Webpack
Split bundles to load only necessary code:
1 module.exports = {2 optimization: {3 splitChunks: {4 chunks: 'all',5 },6 },7 };
4.3 Tree Shaking
Remove unused code:
1 module.exports = {2 mode: 'production',3 };
4.4 Analyze Bundle Size
Install webpack-bundle-analyzer
:
Add to Webpack config:
1 const { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer');23 module.exports = {4 plugins: [new BundleAnalyzerPlugin()],5 };
5. Server-Side Rendering (SSR) and Static Site Generation (SSG)
5.1 Server-Side Rendering with Next.js
Example: SSR with getServerSideProps
1 export async function getServerSideProps() {2 const data = await fetchData();3 return { props: { data } };4 }56 const Page = ({ data }) => <div>{data}</div>;7 export default Page;
5.2 Static Site Generation with Next.js
Example: SSG with getStaticProps
1 export async function getStaticProps() {2 const data = await fetchData();3 return { props: { data } };4 }56 const Page = ({ data }) => <div>{data}</div>;7 export default Page;
6. Image Optimization
6.1 Using next/image
1 import Image from 'next/image';23 const OptimizedImage = () => (4 <Image5 src="/path/to/image.jpg"6 alt="Description"7 width={500}8 height={300}9 priority10 />11 );
6.2 Lazy Loading with Placeholder Images
Install react-lazy-load-image-component
:
Example:
1 import { LazyLoadImage } from 'react-lazy-load-image-component';23 const LazyImage = () => (4 <LazyLoadImage5 alt="Image"6 src="/path/to/image.jpg"7 height="auto"8 width="100%"9 />10 );
7. Conclusion
Optimizing React applications is essential for enhancing performance and the user experience. By leveraging rendering optimizations, virtualization, lazy loading, build improvements, and SSR techniques, developers can create fast and efficient web applications that meet the demands of modern users. Implement these practices in your projects to deliver seamless, high-performance solutions.