Why response.ok in Fetch API Ensures Reliable Code
30 December 20243 min read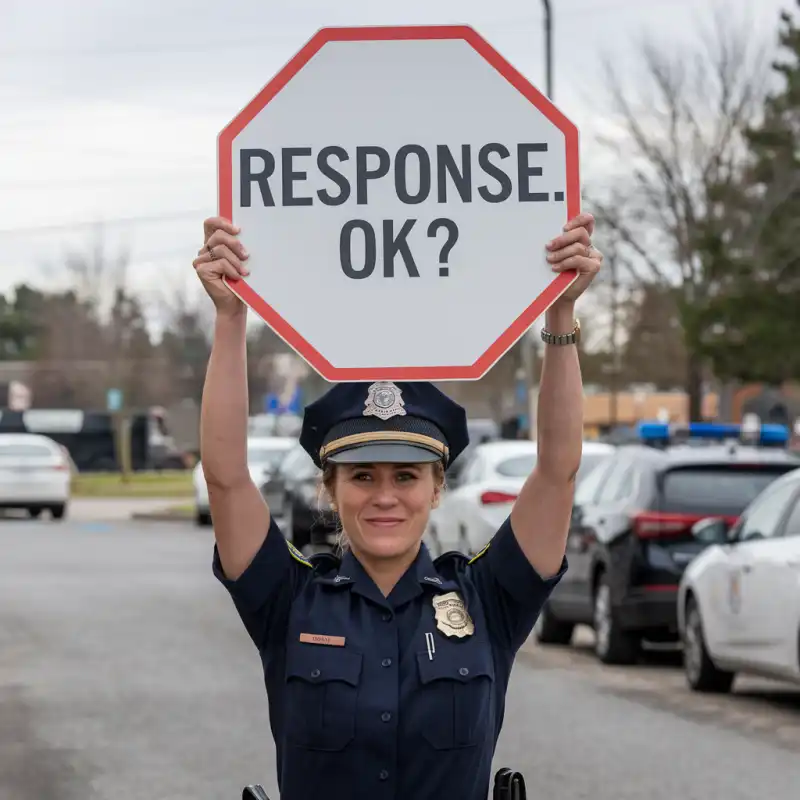
When working with JavaScript's Fetch API, understanding the nuances of HTTP requests and responses is critical for writing robust and reliable code. A common misconception among developers, especially those new to asynchronous programming, is that all errors are caught by the catch
block. This article explains why that's not the case and highlights the importance of checking response.ok
when handling HTTP responses.
Understanding Fetch API Behavior
The Fetch API simplifies making HTTP requests in JavaScript, but its design requires attention to detail. Fetch separates errors into two categories:
- Request Transmission Errors: Issues like network failures or DNS errors occur when the request doesn't reach the server. These errors are caught by the catch block.
- HTTP Response Errors: These happen when the server returns an HTTP status code indicating an error (e.g., 404 or 500). Such responses don't throw exceptions and require manual handling.
Here’s a basic example:
1 fetch(url)2 .then((response) => response.json())3 .catch((error) => console.log(error));
This code only catches transmission errors, not HTTP response errors.
Differentiating Request Transmission from HTTP Response
Request Transmission Success
When a request successfully reaches the server, Fetch considers it a success, regardless of the server’s response status. Errors at this stage, like network issues or invalid URLs, are handled by the catch
block:
1 fetch(url)2 .then((response) => response.json())3 .catch((error) => {4 console.log(error); // Handles issues like network failure or invalid domain5 });
HTTP Response Errors
Even if a server responds with an error status (e.g., 404 Not Found), Fetch treats it as a successful request. For instance:
1 fetch(url)2 .then((response) => {3 console.log(response.status); // Logs 4044 return response.json();5 })6 .catch((error) => console.log(error));
The catch
block won't handle the 404 status because the server returned a response.
The Role of response.ok
The response.ok
property is a boolean that indicates whether the HTTP status code is in the range of 200–299. To handle HTTP errors effectively, you need to check this property:
1 fetch(url)2 .then((response) => {3 if (!response.ok) {4 throw new Error(`HTTP error! Status: ${response.status}`);5 }6 return response.json();7 })8 .catch((error) => console.log(error));
This approach ensures you detect and handle both transmission and response errors.
Common Scenarios Requiring response.ok
Scenario 1: Nonexistent Pages
A request to a non-existent page returns a 404 status:
1 fetch("https://example.com/nonexistent")2 .then((response) => {3 if (!response.ok) {4 throw new Error(`Page not found: ${response.status}`);5 }6 return response.json();7 })8 .catch((error) => console.log(error));
Scenario 2: Unauthorized Access
If access to a resource is forbidden (e.g., 403 status), you can handle it explicitly:
1 fetch("https://example.com/protected")2 .then((response) => {3 if (!response.ok) {4 throw new Error(`Access denied: ${response.status}`);5 }6 return response.json();7 })8 .catch((error) => console.log(error));
Scenario 3: Server Errors
A server-side issue (e.g., 500 Internal Server Error) should also be addressed:
1 fetch("https://example.com/server-error")2 .then((response) => {3 if (!response.ok) {4 throw new Error(`Server error: ${response.status}`);5 }6 return response.json();7 })8 .catch((error) => console.log(error));
Best Practices for Handling Fetch Responses
- Always Check
response.ok
: This ensures you handle HTTP response errors explicitly. - Provide Clear Error Messages: Use descriptive error messages to help debug issues effectively.
- Handle Specific Status Codes: Implement tailored handling for common status codes like 404, 403, and 500.
- Fallback for Invalid JSON: Validate JSON parsing to avoid breaking your application.
Example:
1 fetch(url)2 .then((response) => {3 if (!response.ok) {4 throw new Error(`HTTP error: ${response.status}`);5 }6 return response.json();7 })8 .then((data) => {9 console.log("Data:", data);10 })11 .catch((error) => {12 console.error("Error:", error.message);13 });
Conclusion
Checking response.ok
is a crucial step when working with the Fetch API. It helps you differentiate between request transmission errors and HTTP response errors, making your code more resilient. By explicitly handling various HTTP statuses and providing clear error messages, you can write code that is robust, maintainable, and easier to debug. Start incorporating these best practices today to ensure your applications handle HTTP responses gracefully.