Meet the New Safe Assignment Operator (?=) in JavaScript
Add to your RSS feed14 October 20244 min read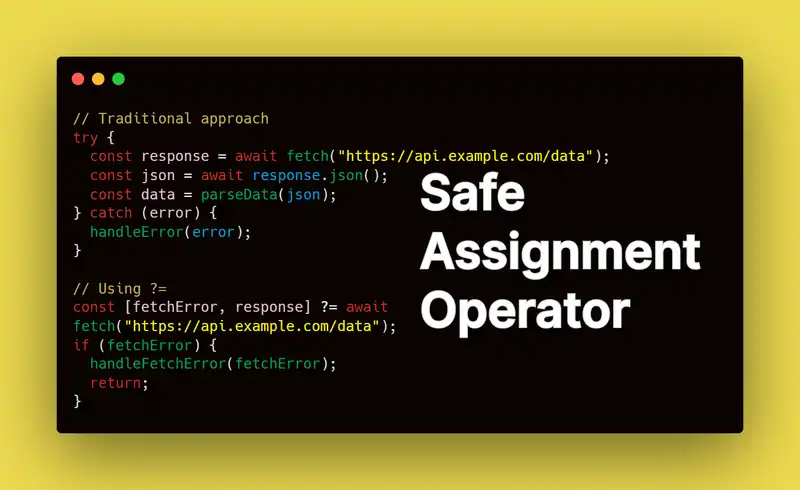
Table of Contents
Modern JavaScript development often encounters tasks involving asynchronous operations and error handling. Typically, try-catch
and async-await
constructs are used for these purposes. However, they can bloat the code and make it harder to read. To address this issue, a new safe assignment operator ?= has been proposed, which significantly simplifies error handling and improves code readability. In this article, we’ll explore how this operator works, its features, and the benefits it offers developers.
Key Features of the ?= Operator:
1. Efficient Error Management
A major benefit of the ?=
operator is its ability to handle errors elegantly and concisely. Instead of wrapping each potentially error-prone operation in try-catch, the safe assignment operator simplifies the process by returning results in the [error, result]
format. If no error occurs, it returns [null, result]
. If an error is present, you get [error, null]
.
1 const [error, data] ?= await fetchData();2 if (error) {3 console.log("Seomething went wrong: ", error);4 } else {5 console.log("Data:", data);6 }
2. Seamless Integration with Async Functions and Promises
The ?=
operator is fully compatible with promises and asynchronous functions, making it ideal for error management in async operations. Whether you’re working with API requests, file handling, or other processes that may fail, this operator simplifies error detection and handling.
1 async function getData() {2 const [fetchError, response] ?= await fetch("https://api.example.com/data");3 if (fetchError) {4 handleFetchError(fetchError);5 return;6 }78 const [jsonError, data] ?= await response.json();9 if (jsonError) {10 handleJsonError(jsonError);11 return;12 }1314 return data;15 }
3. Enhanced Code Readability
The ?=
operator significantly reduces code nesting, eliminates unnecessary try-catch
blocks, and makes error handling more straightforward and intuitive. This leads to cleaner, more maintainable code and minimizes the risk of overlooking important errors.
1 // Traditional approach2 try {3 const response = await fetch("https://api.example.com/data");4 const json = await response.json();5 const data = parseData(json);6 } catch (error) {7 handleError(error);8 }910 // Using ?=11 const [fetchError, response] ?= await fetch("https://api.example.com/data");12 if (fetchError) {13 handleFetchError(fetchError);14 return;15 }
4. Unified Handling
The ?=
operator is compatible with any objects implementing the Symbol.result
method, allowing for a cohesive approach to managing results and errors across different data types and APIs. This flexibility is invaluable when working with complex data structures and various service interactions.
1 const obj = {2 [Symbol.result]() {3 return [new Error("Error"), null];4 },5 };67 const [error, result] ?= obj;8 if (error) {9 console.log("Error:", error);10 }
Understanding Symbol.result
Symbol.result
is a method that can be defined in objects or functions to dictate how results are returned in the context of the safe assignment operator. When invoked with the ?=
operator, the Symbol.result
method processes the returned value, converting it into a user-friendly tuple of error and result: [error, result]
.
For instance, consider an object that implements Symbol.result
to manage its errors:
1 const obj = {2 [Symbol.result]() {3 return [new Error("Object error"), null];4 }5 };67 const [error, result] ?= obj;8 console.log(error); // Outputs: Object error
By supporting Symbol.result
, developers can tailor how objects and functions behave when used with the ?=
operator. This is particularly useful for centralized error and result management across various components of an application. Thus, Symbol.result
standardizes result handling, making code interactions with different APIs and data structures more predictable and consistent.
This mechanism is especially advantageous in libraries or frameworks that require a unified method for error handling. For example, an API request or response object can implement Symbol.result
to return errors or successful data in a consistent format.
With such integration, the ?=
operator enhances not only code readability and structure but also facilitates the development of robust and flexible error-handling solutions.
What is the Purpose of the New Operator?
The main reason for introducing the safe assignment operator is to simplify error handling and enhance code readability. With its streamlined syntax and versatility, the ?=
operator allows for effective management of function execution and asynchronous operations, minimizing the chances of errors and making the code more predictable.
Moreover, using this operator removes the necessity of repeatedly implementing try-catch blocks, which can complicate code, especially in scenarios where a single error might occur at multiple points during program execution. For instance, a data fetch might fail due to network issues (fetch error), during JSON parsing, or while validating the received data. The ?=
operator facilitates handling all these scenarios in a consistent format, thereby simplifying the program structure.
Conclusion
The safe assignment operator ?=
offers developers a novel approach to managing results and errors in JavaScript, making asynchronous processes easier to handle and improving code readability and maintainability. This innovation is especially beneficial for working with promises, APIs, and complex operations where errors may arise at different execution stages.
For detailed information about the proposal and usage examples, check out the GitHub repository.