A Comprehensive Comparison of style.setProperty vs. setAttribute in JavaScript
Add to your RSS feed21 November 20244 min read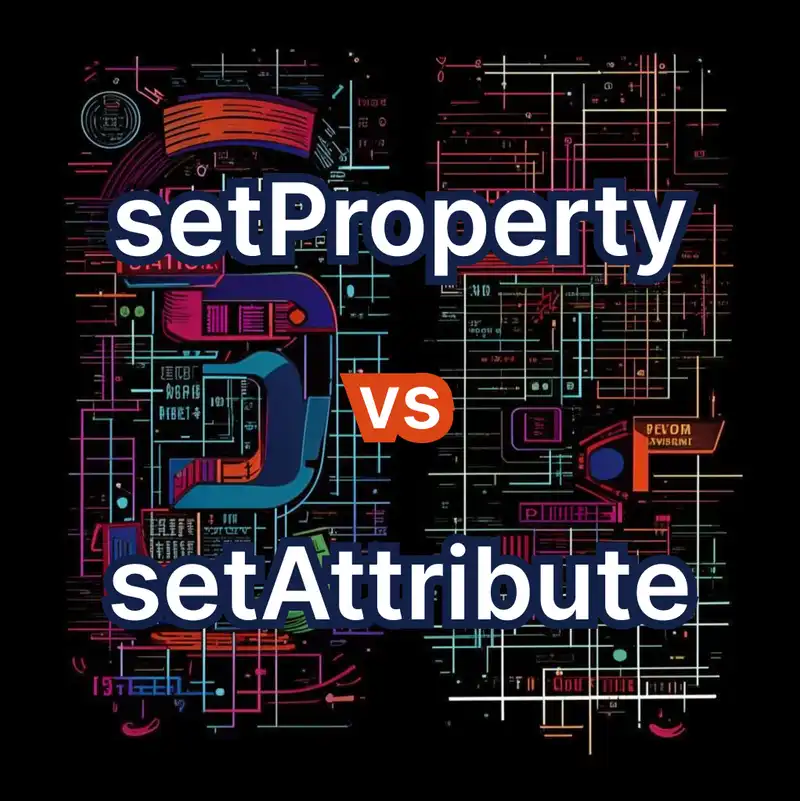
Table of Contents
When working with JavaScript to dynamically manipulate styles, two commonly used methods are style.setProperty
and setAttribute
. Both enable developers to apply styles to HTML elements, but their internal workflows, performance, and use cases differ significantly.
This article combines theoretical exploration, practical analysis, and performance testing to shed light on how these methods function under the hood, particularly in Chromium's Blink engine, and when to use each effectively.
Understanding style.setProperty
and setAttribute
1. The style.setProperty
Method
style.setProperty
directly interacts with the CSS Object Model (CSSOM), the structured representation of CSS in the browser. It validates the property name and value, ensuring correctness before applying the style.
Example:
1 element.style.setProperty("background-color", "red");
This method is ideal when applying specific, validated styles to an element.
2. The setAttribute
Method
setAttribute
operates at the DOM level, setting the style attribute as a single text string. It does not validate the content, allowing invalid or non-standard CSS values to be applied.
Example:
1 element.setAttribute("style", "background-color: red; border: 1px solid blue;");
This method is more suited for applying multiple styles simultaneously or dealing with non-standard attributes.
How Browsers Handle These Methods
1. Internal Workflow of style.setProperty
In Blink (used by Chromium-based browsers), style.setProperty
validates and parses the CSS property and value before integrating them directly into the CSSOM. Here's a breakdown of its workflow:
- Validation: The method checks if the property and value are valid CSS.
- Parsing and Mutation: The value is parsed and added to the CSSStyleDeclaration object associated with the element.
- Performance Overhead: Each property change triggers a process of locking, parsing, and unlocking.
Blink Implementation Snippet:
1 void AbstractPropertySetCSSStyleDeclaration::setProperty(2 const ExecutionContext* execution_context,3 const String& property_name,4 const String& value,5 const String& priority,6 ExceptionState& exception_state) {7 CSSPropertyID property_id = UnresolvedCSSPropertyID(execution_context, property_name);89 if (!IsValidCSSPropertyID(property_id) || !IsPropertyValid(property_id)) {10 return; // Invalid property or value, exit early.11 }1213 SetPropertyInternal(property_id, property_name, value, important, secure_context_mode, exception_state);14 }
Each property is processed independently, meaning multiple calls to style.setProperty
result in repeated parsing and mutation cycles.
2. Internal Workflow of setAttribute
setAttribute
directly modifies the style attribute on the DOM element. Blink processes this by updating the DOM and subsequently parsing the style string into CSSOM properties.
Key steps include:
- Attribute Update: The style attribute is updated with the provided string.
- Parsing: The browser parses the string into individual CSS properties and integrates them into the CSSOM.
- No Validation: Invalid or custom properties are accepted as-is.
Blink Implementation Snippet:
1 void Element::SetAttributeWithValidation(const QualifiedName& name,2 const AtomicString& value,3 ExceptionState& exception_state) {4 SynchronizeAttribute(name);56 AtomicString trusted_value(TrustedTypesCheckFor(7 ExpectedTrustedTypeForAttribute(name), value, GetExecutionContext(),8 "Element", "setAttribute", exception_state));9 if (exception_state.HadException()) {10 return;11 }1213 SetAttributeInternal(FindAttributeIndex(name), name, trusted_value,14 AttributeModificationReason::kDirectly);15 }
This workflow does not validate the content of the style
attribute, allowing for flexibility but also potential inconsistencies.
Performance Testing: style.setProperty
vs. setAttribute
To compare their performance, experiments were conducted under various conditions, such as setting single, multiple, and numeric properties. The results highlight the trade-offs between the two methods.
1. Single Property
Applying a single style property to an element:
1 element.style.setProperty("background-color", "red");
vs
1 element.setAttribute("style", "background-color: red;");
Observation:
style.setProperty
is faster in this case because it directly interacts with the CSSOM, bypassing the need for string parsing. The validation overhead is minimal for single properties.
2. Multiple Properties
Applying multiple styles to an element:
1 element.style.setProperty("background-color", "red");2 element.style.setProperty("border", "1px solid blue");
vs.
1 element.setAttribute("style", "background-color: red; border: 1px solid blue;");
Observation:
setAttribute
performs better when applying multiple styles because the entire style string is parsed and applied in one go. In contrast, style.setProperty
initiates separate parsing and mutation cycles for each property.
3. Numeric Properties
Certain CSS properties (e.g., opacity) accept numeric values directly from JavaScript without requiring string conversion. Testing these properties:
1 element.style.setProperty("opacity", 0.5);
vs.
1 element.setAttribute("style", "opacity: 0.5;");
Observation:
setAttribute
remains faster due to the reduced overhead of handling numeric properties in a single operation. However, the gap between the two methods narrows in this case.
Key Findings
1. Performance Differences:
style.setProperty
is faster for applying a single property.setAttribute
is more efficient for applying multiple styles or dealing with preformatted style strings.
2. Validation:
style.setProperty
validates property names and values, ensuring only valid CSS is applied.setAttribute
does not validate, allowing for greater flexibility but also potential for errors.
3. Use Case Recommendations:
- Use
style.setProperty
when precision and CSS validation are required. - Use
setAttribute
when applying bulk styles or handling custom attributes likedata-
*.
Conclusion
Both style.setProperty
and setAttribute
are valuable tools for CSS manipulation, each suited to specific use cases. By understanding their differences, developers can choose the right method to optimize performance and maintainability in their applications.
- For single or validated property changes,
style.setProperty
is the preferred choice. - For bulk style updates or when working with custom attributes,
setAttribute
offers better efficiency and flexibility.