Improve Prettier Format-On-Save Speed in VS Code
5 November 20243 min read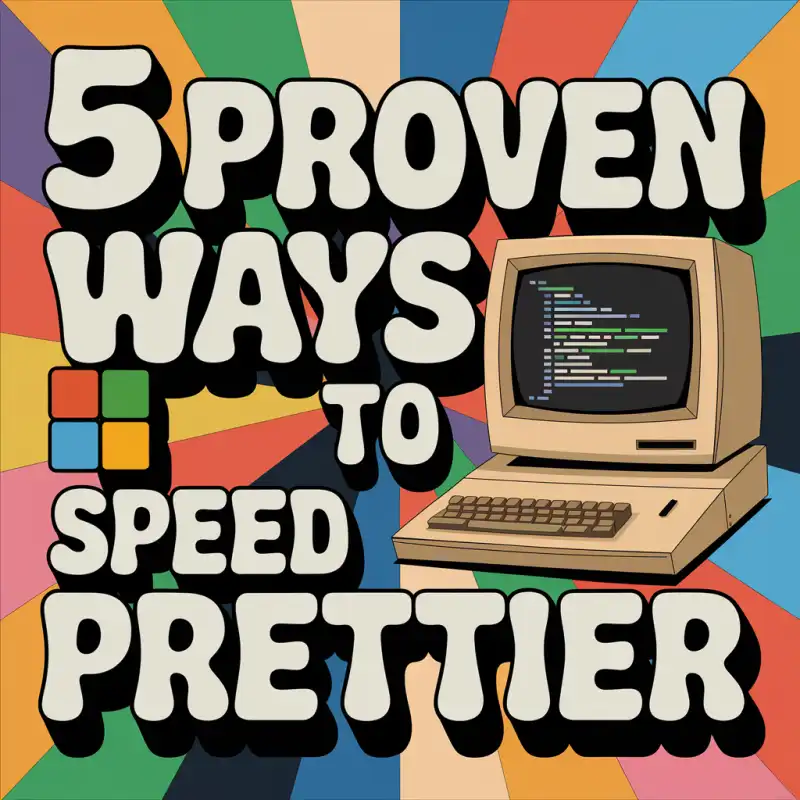
Are you frustrated with slow Prettier formatting when saving files? Many developers face this issue, especially in larger projects. Here's how to significantly improve Prettier's performance without compromising code quality.
1. Enable Format on Save Only for Specific Languages
Instead of formatting every file type, limit Prettier to languages you actually need:
1 {2 "[javascript]": {3 "editor.formatOnSave": true,4 "editor.defaultFormatter": "esbenp.prettier-vscode"5 },6 "[typescript]": {7 "editor.formatOnSave": true,8 "editor.defaultFormatter": "esbenp.prettier-vscode"9 },10 // Disable for other file types11 "[json]": {12 "editor.formatOnSave": false13 }14 }
2. Use .prettierignore
Effectively
Create a .prettierignore
file in your project root to skip unnecessary files:
1 # Dependencies2 node_modules3 **/node_modules4 package-lock.json56 # Build outputs7 dist8 build9 coverage10 .next1112 # Large generated files13 *.min.js14 *.bundle.js1516 # Other files you rarely edit17 *.svg18 *.png
3. Configure Prettier for Performance
Add these settings to your .prettierrc
:
1 {2 "printWidth": 100,3 "useTabs": false,4 "tabWidth": 2,5 "requirePragma": true,6 "insertPragma": true7 }
The requirePragma
option is particularly important - it tells Prettier to only format files that have a special comment at the top:
1 /** @format */
4. Use Editor Config for Better Performance
Create an .editorconfig
file to handle basic formatting, reducing Prettier's workload:
1 root = true23 [*]4 indent_style = space5 indent_size = 26 end_of_line = lf7 charset = utf-88 trim_trailing_whitespace = true9 insert_final_newline = true
5. Optimize VS Code Settings
Update your VS Code settings to improve formatting performance:
1 {2 "editor.formatOnSaveTimeout": 5000,3 "editor.formatOnSave": true,4 "editor.formatOnPaste": false,5 "editor.formatOnType": false,6 "files.autoSave": "onFocusChange"7 }
Additional Performance Tips
- Use Latest Versions: Keep Prettier and the VS Code extension updated for latest performance improvements.
- Consider Using prettier-eslint: If you're using ESLint, integrate it with Prettier to avoid double processing:
npm install --save-dev prettier-eslint
- Format Selected Files Only: Instead of formatting the entire project, use VS Code's file explorer to selectively format files or directories.
- Use Git Pre-commit Hooks: Move formatting to pre-commit hooks using husky and pretty-quick:
npm install --save-dev husky pretty-quickAdd to your package.json:
1 {2 "husky": {3 "hooks": {4 "pre-commit": "pretty-quick --staged"5 }6 }7 }
5. Remove the Auto Import
plugin and similar extensions to streamline your development environment.
These plugins can sometimes introduce unnecessary complexity or slow down your workflow. By uninstalling them, you can enhance the performance of your IDE and improve your coding experience.
Common Issues and Solutions
1. Slow on Large Files:
- Split large files into smaller modules
- Increase
formatOnSaveTimeout
in VS Code settings
2. Conflicts with ESLint:
- Use
eslint-config-prettier
to disable conflicting rules - Configure ESLint to run after Prettier
3. High CPU Usage:
- Limit the number of file watchers
- Use
requirePragma
option
Using VS Code's Extension Bisect to Identify Plugin Conflicts with Prettier
VS Code’s Extension Bisect feature is designed to pinpoint extensions causing problems by automatically disabling and enabling them until it finds the conflicting extension. Here’s a quick guide on using it:
- Activate Extension Bisect: Press
Cmd+Shift+P
(Mac) orCtrl+Shift+P
(Windows/Linux) and type “Start Extension Bisect.” VS Code will begin disabling half of your extensions. - Reproduce the Issue: Test Prettier’s format-on-save functionality. If the problem persists, Extension Bisect will continue testing the remaining extensions until it identifies the culprit.
- Identify and Resolve: Once the conflicting extension is found, VS Code will inform you. You can choose to uninstall or disable the problematic plugin.
This method simplifies troubleshooting by automating the process of identifying which extension conflicts with Prettier, helping you restore smooth operation without manually testing each one.
Conclusion
By implementing these optimizations, you can significantly reduce Prettier's formatting time. Start with the most impactful changes like proper .prettierignore configuration and language-specific formatting, then fine-tune with additional optimizations as needed.
Remember, the goal is to find the right balance between code formatting and development speed. Not every file needs to be formatted on every save, and not every project requires the same level of formatting rigor.