Tracking Points in JavaScript for Monitoring Purposes
24 October 202416 min read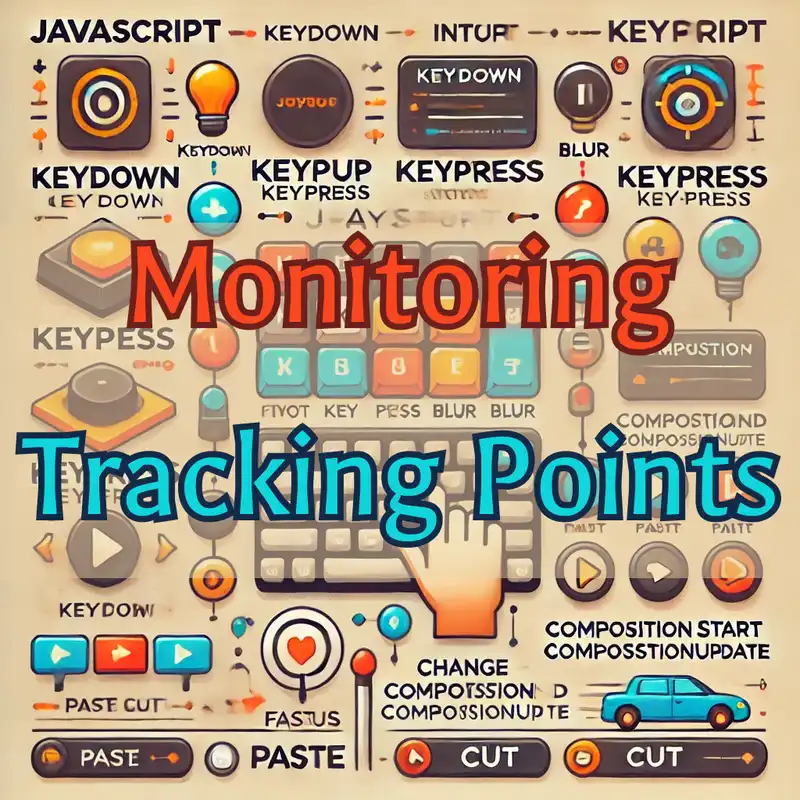
Here’s an explanation of how to implement and use monitoring tracking points in JavaScript to track and monitor specific actions, events, or performance metrics in web applications.
What Are Monitoring Tracking Points?
Monitoring tracking points in JavaScript refer to strategically placed code snippets that help track user interactions, system performance, or any other significant events within a web application. These points can monitor things like page views, button clicks, API response times, and other key user actions or performance metrics. By adding tracking points, you can collect data that improves user experience and helps debug or optimize performance.
Implementation of Monitoring Tracking Points in JavaScript
You can implement monitoring tracking points using various tools like logging services, custom event listeners, or third-party libraries such as Google Analytics or Sentry for performance monitoring and error tracking.
Here’s a breakdown of how to set up tracking points in JavaScript.
1. Tracking User Events (Click, Scroll, etc.)
A common use case is tracking user interactions, such as clicks, form submissions, or page scrolls.
1 // Function to log or send tracking data to a server2 function trackEvent(eventType, details) {3 console.log(`Event: ${eventType}`, details);4 // Optionally send data to an analytics service5 // fetch('/tracking-endpoint', { method: 'POST', body: JSON.stringify({ eventType, details }) });6 }78 // Example: Track button click events9 const button = document.getElementById('myButton');10 button.addEventListener('click', function () {11 trackEvent('button_click', { buttonId: 'myButton', timestamp: Date.now() });12 });1314 // Example: Track page scroll15 window.addEventListener('scroll', function () {16 trackEvent('page_scroll', { scrollY: window.scrollY, timestamp: Date.now() });17 });
2. Performance Monitoring
JavaScript provides the Performance
API, which allows you to measure how long certain operations take. You can track performance metrics like page load times, API response times, and rendering times.
1 // Example: Measure page load time2 window.addEventListener('load', function () {3 const pageLoadTime = performance.now();4 trackEvent('page_load', { duration: pageLoadTime });5 });67 // Example: Measure how long an API call takes8 function measureApiCallPerformance() {9 const start = performance.now();1011 fetch('https://api.example.com/data')12 .then(response => response.json())13 .then(data => {14 const duration = performance.now() - start;15 trackEvent('api_call', { duration: duration, endpoint: 'https://api.example.com/data' });16 });17 }
3. Error Tracking
You can track JavaScript errors that occur in your application using the window.onerror
event or by using libraries like Sentry for more detailed tracking.
1 // Basic error tracking using window.onerror2 window.onerror = function (message, source, lineno, colno, error) {3 trackEvent('js_error', {4 message: message,5 source: source,6 lineno: lineno,7 colno: colno,8 error: error ? error.stack : ''9 });10 };1112 // Example: Using a third-party service like Sentry for error tracking13 Sentry.init({ dsn: 'https://examplePublicKey@o0.ingest.sentry.io/0' });14 Sentry.captureException(new Error('Something went wrong'));
4. Custom Metrics Tracking
Sometimes you may want to track custom metrics such as specific user actions or feature usage. You can send this data to an analytics service or store it locally.
1 // Custom metric: Track time spent on a specific section of the page2 let sectionStartTime = 0;3 const sectionElement = document.getElementById('sectionId');45 sectionElement.addEventListener('mouseenter', function () {6 sectionStartTime = Date.now();7 });89 sectionElement.addEventListener('mouseleave', function () {10 const timeSpent = Date.now() - sectionStartTime;11 trackEvent('time_spent', { section: 'sectionId', duration: timeSpent });12 });
Using Third-Party Tools for Monitoring and Tracking
For more advanced monitoring, you can integrate with third-party tools like Google Analytics, Mixpanel, or Sentry. These tools provide robust tracking solutions for user activity, performance monitoring, and error logging.
Example: Integrating with Google Analytics
1 // Tracking a custom event in Google Analytics2 function trackCustomEvent(category, action, label, value) {3 gtag('event', action, {4 'event_category': category,5 'event_label': label,6 'value': value7 });8 }910 // Example: Track a button click in Google Analytics11 const trackableButton = document.getElementById('trackButton');12 trackableButton.addEventListener('click', function () {13 trackCustomEvent('Button', 'Click', 'Track Button', 1);14 });
Example: Using Sentry for Error Tracking
1 // Initialize Sentry for error monitoring2 Sentry.init({3 dsn: 'https://examplePublicKey@o0.ingest.sentry.io/0'4 });56 // Capture a custom error7 try {8 // Code that may throw an error9 } catch (error) {10 Sentry.captureException(error);11 }
Browser Window Events
Browser window events are important for responding to changes in the state of the browser window. They allow developers to create responsive web applications that adapt to user actions and changes in the environment.
Types of Browser Window Events
- resize: Fired when the browser window is resized.
- scroll: Fired when the user scrolls the document or an element.
- load: Fired when the entire page (including all dependent resources) has finished loading.
- unload: Fired when the document is about to be unloaded (e.g., navigating away from the page).
- beforeunload: Fired before the user leaves the page, allowing for a confirmation dialog.
- focus: Fired when the window gains focus.
- blur: Fired when the window loses focus.
Event Properties
- event.target: The window object that triggered the event.
- event.preventDefault(): Prevents the default action of the event (e.g., preventing navigation).
- window.innerWidth: The width of the window's content area.
- window.innerHeight: The height of the window's content area.
Example
1 window.addEventListener('resize', function() {2 console.log(`Window resized to ${window.innerWidth} x ${window.innerHeight}`);3 });
Common Mouse Events
Common mouse events are essential for capturing user interactions with various elements on a webpage. These events enhance the interactivity of web applications and allow developers to create engaging user experiences.
Types of Common Mouse Events
- click: Fired when the mouse button is clicked on an element.
- dblclick: Fired when the mouse button is double-clicked on an element.
- mousedown: Fired when a mouse button is pressed down on an element.
- mouseup: Fired when a mouse button is released over an element.
- mouseover: Fired when the mouse pointer enters the area of an element.
- mouseout: Fired when the mouse pointer leaves the area of an element.
- mousemove: Fired continuously as the mouse pointer moves within the area of an element.
Event Properties
- event.clientX: Returns the horizontal coordinate of the mouse pointer relative to the viewport.
- event.clientY: Returns the vertical coordinate of the mouse pointer relative to the viewport.
- event.button: Indicates which mouse button was pressed (0: left, 1: middle, 2: right).
- event.target: The element that triggered the event.
Example
1 document.addEventListener('click', function(event) {2 console.log(`Mouse clicked at (${event.clientX}, ${event.clientY})`);3 });
Keyboard Events
Keyboard events are important for handling user interactions via the keyboard. These events allow developers to respond to key presses and releases, enabling rich user experiences in web applications.
Types of Keyboard Events
- event.key: Returns the value of the key pressed.
- event.code: Returns the physical key on the keyboard.
- event.altKey: Indicates if the Alt key was pressed.
- event.ctrlKey: Indicates if the Ctrl key was pressed.
- event.shiftKey: Indicates if the Shift key was pressed.
Example
1 document.addEventListener('keydown', function(event) {2 if (event.key === 'Enter') {3 console.log('Enter key was pressed!');4 }5 });
Form Events
Form events are essential for handling user interactions with forms in web applications. They enable developers to validate input, manage submissions, and provide feedback to users.
Types of Form Events
- submit: Fired when a form is submitted.
- input: Fired when an input or textarea value changes.
- change: Fired when the value of an input, select, or textarea changes and loses focus.
- focus: Fired when an element gains focus.
- blur: Fired when an element loses focus.
Event Properties
- event.target: The element that triggered the event.
- event.preventDefault(): Prevents the default action of the event (e.g., submitting a form).
- event.stopPropagation(): Stops the event from bubbling up to parent elements.
Example
1 document.querySelector('form').addEventListener('submit', function(event) {2 event.preventDefault(); // Prevent the form from submitting3 console.log('Form submitted!');4 });
Complete Tracking Example: Monitoring Page Views, Button Clicks, and Form Submissions
This example demonstrates how to track page views, button clicks, and form submissions on your website. The approach involves identifying specific elements or events to track and sending the data to a server.
Step 1: Identify Elements and Events to Track in HTML
In the HTML below, we define a button and a form with custom data-track-id
attributes to uniquely identify them:
1 <button id="myButton" data-track-id="button1">Click Me</button>23 <form id="myForm">4 <input type="text" name="username" placeholder="Username">5 <input type="password" name="password" placeholder="Password">6 <button type="submit">Submit</button>7 </form>
Here, the data-track-id
attribute is added to identify the button and form elements for tracking purposes.
Step 2: Monitor Events and Capture Event Data Using JavaScript
Now, we use JavaScript to listen for events like page load, button click, and form submission, then capture relevant event data.
1 // Listen for page load event2 window.addEventListener("load", function() {3 const pageUrl = window.location.href;4 const timestamp = new Date().getTime();5 const userData = {6 eventType: "pageView",7 pageUrl: pageUrl,8 timestamp: timestamp9 // Add any other relevant user data10 };1112 // Send the data to the server13 sendData(userData);14 });1516 // Listen for button click event17 document.getElementById("myButton").addEventListener("click", function(event) {18 const buttonId = event.target.getAttribute("data-track-id");19 const timestamp = new Date().getTime();20 const userData = {21 eventType: "buttonClick",22 buttonId: buttonId,23 timestamp: timestamp24 // Add any other relevant user data25 };2627 // Send the data to the server28 sendData(userData);29 });3031 // Listen for form submission event32 document.getElementById("myForm").addEventListener("submit", function(event) {33 event.preventDefault(); // Prevent default form submission3435 const formId = event.target.getAttribute("id");36 const formData = new FormData(event.target);37 const timestamp = new Date().getTime();38 const userData = {39 eventType: "formSubmit",40 formId: formId,41 formData: Object.fromEntries(formData.entries()), // Convert form data to JSON42 timestamp: timestamp43 // Add any other relevant user data44 };4546 // Send the data to the server47 sendData(userData);48 });
This JavaScript code listens for specific events like page load, button click, and form submission. It gathers event data, such as page URL, button ID, form ID, and form field values, and prepares it for transmission.
Step 3: Send Event Data to the server
1 function sendData(data) {2 fetch("/track", {3 method: "POST",4 headers: {5 "Content-Type": "application/json"6 },7 body: JSON.stringify(data)8 })9 .then(response => {10 if (response.ok) {11 console.log("Data sent successfully.");12 } else {13 console.error("Error sending data.");14 }15 })16 .catch(error => {17 console.error("Network error:", error);18 });19 }
In this sendData()
function, we use the fetch
API to send a POST request to the /track
endpoint on the server. The event data is sent as JSON in the request body.
This implementation shows how to efficiently track key user interactions such as page views, button clicks, and form submissions using modern JavaScript.
IntersectionObserver for Monitoring Tracking Points
The IntersectionObserver
API is a powerful tool for monitoring when elements enter or leave the viewport, making it ideal for setting up tracking points in JavaScript. You can use it to track user interactions, element visibility, lazy-loading images, or triggering animations when an element appears on the screen. Here's a guide on how to use the IntersectionObserver
for monitoring tracking points.
What is the IntersectionObserver?
The IntersectionObserver
API allows you to asynchronously observe changes in the intersection of a target element with a root element (typically the viewport). This makes it useful for detecting when elements become visible (or invisible) to the user, which can be leveraged to monitor user engagement or track metrics like viewability.
Basic Usage of IntersectionObserver
1. Creating an IntersectionObserver
To create an IntersectionObserver
, you need to define a callback function that will be triggered when the target element's visibility changes. You also specify options such as thresholds (which defines when the callback is triggered) and the root (which defines the container to observe within).
Here’s a basic implementation:
1 // Callback function that handles intersection changes2 function handleIntersection(entries, observer) {3 entries.forEach(entry => {4 if (entry.isIntersecting) {5 // Element has entered the viewport6 console.log('Element is visible:', entry.target);78 // You can track the event here9 trackEvent('element_visible', { elementId: entry.target.id });1011 // Optionally, stop observing the element after it is visible12 observer.unobserve(entry.target);13 }14 });15 }1617 // Create an IntersectionObserver instance18 const observer = new IntersectionObserver(handleIntersection, {19 root: null, // Use the viewport as the container20 threshold: 0.5 // Trigger when 50% of the element is visible21 });2223 // Observe an element24 const targetElement = document.getElementById('target');25 observer.observe(targetElement);2627 // Example tracking function28 function trackEvent(eventType, details) {29 console.log(`Tracked event: ${eventType}`, details);30 // Send tracking data to your server or analytics service here31 }
Explanation
- Callback (handleIntersection): This function is called whenever the target element’s visibility changes. Each
entry
inentries
contains information about an observed element, including entry.isIntersecting, which indicates whether the element is in the viewport.
Observer options:
- root: Defines the element that is used as the viewport for checking visibility. Setting it to null means the actual browser viewport.
- threshold: Defines how much of the target element should be visible before triggering the callback. For example, a threshold of
0.5
means the callback will trigger when 50% of the element is visible.
2. Tracking Multiple Elements
You can easily observe multiple elements with the same IntersectionObserver
instance.
1 // Target multiple elements for tracking2 const elementsToTrack = document.querySelectorAll('.trackable');34 // Observe each element5 elementsToTrack.forEach(element => {6 observer.observe(element);7 });
This will monitor visibility for all elements with the .trackable
class. You can then track metrics like how often certain sections are viewed.
Lazy Loading Images Using IntersectionObserver
One common use case for IntersectionObserver
is lazy-loading images—loading images only when they come into view, reducing initial page load times.
1 // Callback for lazy-loading images2 function lazyLoadImages(entries, observer) {3 entries.forEach(entry => {4 if (entry.isIntersecting) {5 const img = entry.target;6 img.src = img.dataset.src; // Replace placeholder with the actual image source7 observer.unobserve(img); // Stop observing the image once it’s loaded8 }9 });10 }1112 // Create an observer for lazy loading13 const imageObserver = new IntersectionObserver(lazyLoadImages, { threshold: 0.1 });1415 // Observe all images with the data-src attribute16 document.querySelectorAll('img[data-src]').forEach(img => {17 imageObserver.observe(img);18 });
Advanced Use Cases
Tracking Scroll Depth
IntersectionObserver
can also be used to track how far users scroll down a page. For example, you can place invisible tracking points at specific sections (e.g., 25%, 50%, 75%, and 100%) of your content.
1 // Create scroll depth tracking points2 const scrollTrackingPoints = document.querySelectorAll('.scroll-depth');34 // Create observer to track scroll depth5 const scrollObserver = new IntersectionObserver((entries) => {6 entries.forEach(entry => {7 if (entry.isIntersecting) {8 // Log or send scroll depth event9 trackEvent('scroll_depth', { section: entry.target.dataset.section });10 scrollObserver.unobserve(entry.target); // Track each point only once11 }12 });13 }, { threshold: 0.5 });1415 // Observe each scroll depth marker16 scrollTrackingPoints.forEach(point => scrollObserver.observe(point));
Customizing the Root and Threshold
The IntersectionObserver
can be customized further by changing the root element (not just the viewport) and adjusting the threshold for triggering the callback. For example, tracking visibility within a specific container.
1 // Create observer with custom root element and multiple thresholds2 const container = document.querySelector('.scrollable-container');3 const observer = new IntersectionObserver(handleIntersection, {4 root: container, // Observe within this container5 threshold: [0, 0.25, 0.5, 0.75, 1] // Track different visibility percentages6 });
Best Practices for Using IntersectionObserver
- Stop Observing Elements: Once you no longer need to track an element (e.g., it’s already been tracked or the element is removed), use observer.unobserve(element) to stop observing it and improve performance.
- Threshold Selection: Choose appropriate thresholds based on the interaction you’re monitoring. For viewability tracking, values like 0.25 or 0.5 are common. For animations, a threshold of 0 might be enough.
- Fallback for Older Browsers: Ensure that you have a fallback for older browsers that don’t support IntersectionObserver (e.g., use polyfills).
The IntersectionObserver
API is a powerful and efficient way to monitor elements for visibility and track user interactions in JavaScript. Whether you’re using it for lazy loading, scroll tracking, or triggering events when elements come into view, it can significantly improve performance and help you gather important metrics with minimal overhead.
With this API, you can easily implement monitoring tracking points to better understand user engagement, optimize your app, and create a more dynamic and responsive user experience.
Using MutationObserver for Monitoring Tracking Points
The MutationObserver
API allows you to monitor changes to the DOM in real-time. It’s useful for tracking changes to specific elements, such as when a user interacts with a page or when elements are dynamically added or removed. This example will demonstrate how to use MutationObserver to track certain DOM mutations like attribute changes, added/removed nodes, or text content updates.
Step 1: Identify Elements to Monitor in HTML
Here’s a simple HTML structure where we track changes to a div element that will dynamically update:
1 <div id="trackingDiv" data-track-id="div1">Track changes in this element</div>23 <button id="updateButton">Update Element</button>
Step 2: Use JavaScript to Set Up MutationObserver
We will use MutationObserver
to watch for changes in the div
element, specifically monitoring changes in the attributes, child nodes, or text content.
1 // Target element to observe2 const targetNode = document.getElementById('trackingDiv');34 // MutationObserver callback function5 const observerCallback = (mutationsList, observer) => {6 mutationsList.forEach(mutation => {7 if (mutation.type === 'childList') {8 console.log('Child nodes changed:', mutation);9 } else if (mutation.type === 'attributes') {10 console.log('Attributes changed:', mutation);11 } else if (mutation.type === 'characterData') {12 console.log('Text content changed:', mutation);13 }1415 // Send mutation data for tracking purposes16 const mutationData = {17 mutationType: mutation.type,18 targetElement: mutation.target.id || mutation.target.tagName,19 changedAttribute: mutation.attributeName || null,20 addedNodes: mutation.addedNodes.length ? mutation.addedNodes : null,21 removedNodes: mutation.removedNodes.length ? mutation.removedNodes : null22 };2324 // Send mutation data to server25 sendData(mutationData);26 });27 };2829 // Set up the observer options (track attributes, child nodes, and text content)30 const observerOptions = {31 attributes: true,32 childList: true,33 subtree: true,34 characterData: true35 };3637 // Initialize the MutationObserver38 const observer = new MutationObserver(observerCallback);3940 // Start observing the target node41 observer.observe(targetNode, observerOptions);4243 // Example: Simulate updates with a button click44 document.getElementById('updateButton').addEventListener('click', () => {45 // Modify the target element's content46 targetNode.innerHTML = '<p>New content added</p>';47 targetNode.setAttribute('data-new-attribute', 'newValue');48 });
Step 3: Send Mutation Data to the Server
Just like with tracking other events, we will use the fetch
API to send mutation data to the server:
1 function sendData(data) {2 fetch("/track", {3 method: "POST",4 headers: {5 "Content-Type": "application/json"6 },7 body: JSON.stringify(data)8 })9 .then(response => {10 if (response.ok) {11 console.log("Mutation data sent successfully.");12 } else {13 console.error("Error sending mutation data.");14 }15 })16 .catch(error => {17 console.error("Network error:", error);18 });19 }
How It Works:
- The MutationObserver monitors the targetNode for any changes in its attributes, child elements, and text content.
- When a mutation occurs, the callback function processes the mutation and sends the relevant data to a server using fetch.
- This method can track dynamic changes in real-time, providing insights into how users interact with or modify the DOM, which can be useful for tracking user behavior, performance monitoring, or debugging.
This approach provides a powerful way to track DOM mutations dynamically, such as changes in user interface elements, content updates, or attribute modifications, allowing for real-time analytics and monitoring on your web pages.
Conclusion
Monitoring tracking points in JavaScript can be implemented in a variety of ways to track user behavior, measure performance, and detect errors. Whether using custom event listeners or integrating with third-party services, these tracking points are essential for improving user experience and ensuring application reliability. By collecting and analyzing this data, you can make data-driven decisions to enhance your application’s performance and functionality.