Mastering useCombinedRef React Hook
6 February 20257 min read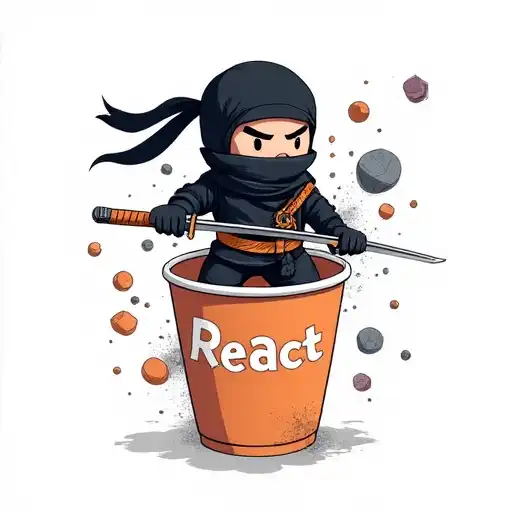
When developing in React, direct interaction with DOM elements is often necessary. React provides the refs mechanism to access elements after rendering. The most common approach is using object refs via useRef
, but another option is callback refs, which offer greater flexibility and control over the lifecycle of elements. This article explains how callback refs work, their advantages, common issues, and practical use cases.
How Callback Refs Work
Callback refs provide a more precise way to manage ref bindings compared to object refs. Here's how they function:
- Mounting: When an element is mounted in the DOM, React calls the ref function with the element itself, allowing immediate interaction.
- Unmounting: When an element is removed, React calls the ref function with null, enabling cleanup operations.
Example: Tracking Mounting and Unmounting
In this example, the handleRef
callback logs the element when it mounts and unmounts. The isVisible
state toggles the visibility of the tracked element. Whenever the element appears or disappears, handleRef
is called, allowing us to track its lifecycle.
Common Issues and Solutions
Issue: Unnecessary Callback Ref Calls
A common problem is that React recreates the ref function on every re-render, leading to unwanted ref reassignments. This happens because React thinks it's dealing with a new ref, triggering a null
call before assigning the new node.
Solution: Memoizing with useCallback
We can avoid this by using useCallback
to ensure the function remains unchanged unless dependencies change. This memoization prevents unnecessary ref calls and maintains the correct ref binding.
This ensures that refCallback is created only once and prevents unnecessary reassignments. The ref function remains stable across re-renders, maintaining the correct ref binding.
Callback Refs vs useEffect
and useLayoutEffect
Callback refs interact differently with useEffect
and useLayoutEffect
:
- Callback refs fire before
useLayoutEffect
anduseEffect
. useLayoutEffect
runs after DOM updates but before the browser repaints.useEffect
runs after the browser has painted.
Console Output Order:
"Callback ref assigned: [div element]"
"useLayoutEffect triggered"
"useEffect triggered"
Solving Issues with Dynamic Elements
Problem: useRef
Fails with Changing Elements
Using useRef
with elements that dynamically change (e.g., swapping a <div>
for a <p>
) can cause issues because useRef holds onto the old element reference.
Here, when toggling elements, ResizeObserver
may still track the removed element.
Solution: Using Callback Refs
Callback refs ensure proper reassignment when the element changes.
Here, the observer automatically attaches/detaches as elements change. The useResizeObserver
hook manages the ResizeObserver lifecycle, ensuring it tracks the correct element.
Combining Multiple Refs
Callback refs can also help when multiple refs need to be merged.
This allows both internal logic and external props-based refs to function correctly.
React 19 Updates
React 19 improves callback refs by automatically cleaning up old references, simplifying code.
When to Use Callback Refs
- Use
useRef
for simple element access. - Use callback refs for complex lifecycle control, reusable hooks, or dynamic elements.
Optimized useCombinedRef
Hook
Below is an optimized implementation of useCombinedRef
, improving readability, performance, and maintainability.
Advantages of Using useCombinedRef
1. Supports Multiple Ref Types
- Works with both callback refs and object refs without conflicts.
- Ensures seamless integration with various ref-based APIs.
2. Improved Code Readability and Reusability
- The
useCombinedRef
function can be reused across multiple components. - Reduces redundant logic, keeping components clean and maintainable.
3. Prevents Unnecessary Re-Renders
- Uses
useCallback
to memoize the function, avoiding ref resets on re-renders. - Keeps reference handling stable for optimal performance.
4. Automatic Resource Management
- Ensures old references are properly updated or cleaned up.
- Helps prevent memory leaks in complex component structures.
5. Versatility in Component Design
- Useful for library authors and UI framework developers.
- Ensures existing logic remains intact while adding ref-based functionality.
Conclusion
Using useCombinedRef
provides a cleaner, more efficient approach to handling multiple refs in React components. Whether you're building reusable UI components or integrating third-party libraries, this hook ensures seamless ref management without unnecessary complexity. 🚀